Introduction
After successfully executing the Individual Health Identifier (IHI) Lookup, it is important to modify your implementation to meet the Test Cases for the Use Cases we have built to.
- UC.010 (adding a new patient in your local system and retrieving their IHI), and
- UC.015 (updating patient details in your local system and revalidating their IHI).
Both of these Use Cases are very similar and utilise the same Healthcare Identifiers (HI) Service web service.
Important!
Please review the UC.010 and UC.015 sections in the HI Service Conformance Test Specification document now:
Healthcare Identifiers Service - Support Documents - Conformance Test Specification v3.4
Important!
Note that there are more Use Cases for IHIs however the most common are UC.010 and UC.015. Refer to the Conformance Test Specification link above for more information.
Use Case 010 (UC.010) – Register Patient
Use Case Name | Register Patient |
Purpose | Create a new patient health record. |
Outline | A new patient health record is created. If possible an IHI is obtained from the HI Service and associated with the record. |
Sample of Test Cases covered in this page | HI_010_005805, HI_010_005808, HI_010_005817 |
Clarification
The ‘Register Patient’ Use Case (UC.010) involves adding a new patient to your local system (e.g. a new patient attends a practice where an administrator creates a new patient record). It does not mean registering the patient for an IHI in the HI service.
We will now walk through several Test Cases for UC.010. The first few examples are introductory to give you a feel for the test cases. Please be sure to review all the applicable Test Cases for each Use Case.
Test Case ID | HI_010_005805 |
---|---|
Test Objective | When interacting with the HI Service the software shall be able to send no more than 40 characters for a patient's family name and send no more than 40 characters for a patient's given name. The given and family names shall be stored in full in the software system. If the HI Service returns a shortened patient name then the local system shall ensure the shortened name does not replace the full length patient name. |
How to evaluate | Perform a patient health record registration operation such that the software system interacts with the HI Service. If local system allows, enter more than 40 characters for a patient's family name and more than 40 characters for a patient's given name. a. Verify that the software sends no more than 40 characters for a patient's family name |
Code Example
The following functionality could be applied to meet this test case. This code can be used as a guide to assist you when sending a family name and given name for an IHI lookup.
string familyName = "Name with More than 40 characters should send only 40 characters.";
request.familyName = familyName.Substring(0, 40);
string givenName = "Name with More than 40 characters should send only 40 characters.";
request.givenName = givenName.Substring(0, 40);
Test Case ID | HI_010_005808 |
---|---|
Test Objective | The software shall allow for the capture and storage of a patient’s full date of birth inclusive of day, month and four-digit year. |
How to evaluate | a. Verify that software prevents the capture of a date of birth without the day value, month value, and year value. b. Verify that software prevents the capture and storage of a date of birth with the year value less or greater than four digits. c. Verify that software allows the capture of a date of birth inclusive of day, month and 4-digit year. |
Code Example
The following code example shows a method to validate the date of birth when entered manually. Your software likely already conducts similar validation.
public static bool IsValidDate(int year, int month, int day)
{
if (year < DateTime.MinValue.Year || year > DateTime.MaxValue.Year)
return false;
if (month < 1 || month > 12)
return false;
return day > 0 && day <= DateTime.DaysInMonth(year, month);
}
UI Example 1: Calendar control, where users automatically select valid date of birth that includes year, month and day.
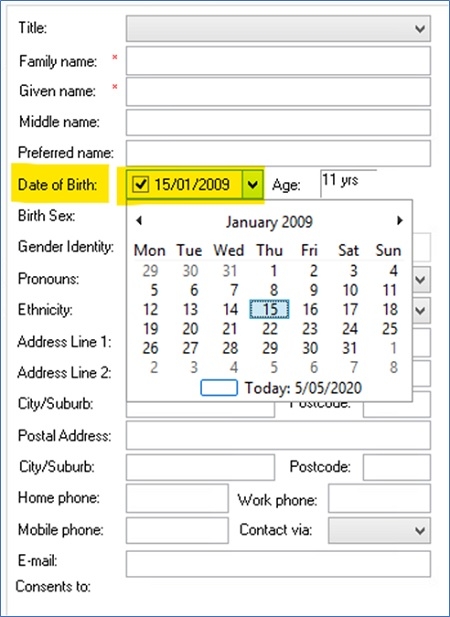
UI Example 2: Individual dropdown controls for the day, month and year.
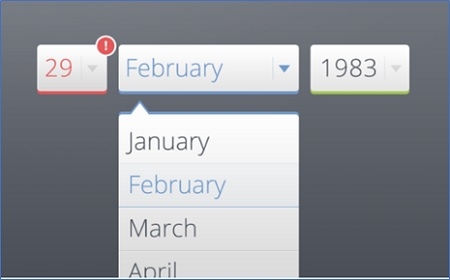
Test Case ID | HI_010_005820 |
---|---|
Test Objective | When assigning a new IHI or updating IHI details in a patient record, the software shall store the following: - the IHI number; |
How to evaluate | Assign a new IHI to a patient health record that has an IHI number. a. Verify that software stores the IHI number. b. Verify that software stores the IHI number status (Active/Deceased/Retired/Expired/Resolved). c. Verify that software stores the date and time of the assignment/update d. Verify that software records the current IHI record status (Verified/Unverified/Provisional). |
Code Example
The following code example demonstrates how you can save and update the patient record with the mandatory IHI details. We have used dummy classes and objects (Patient, HIResponse, HIHelperService, etc) to demonstrate this functionality.
When the IHI status is “Active” or “Deceased” the response is simple to interpret as they are returned in the <ihiStatus> tag. However, when the patient IHI status is “Retired”, “Expired” or “Resolved” it is not returned within the <ihiStatus> tag and the response will be returned with a service message as shown in the table below.
Please use the test data to see the different responses from the HI Service.
IHI Status | Code | Description |
---|---|---|
Expired | 01613 | Error: The IHI Number will not be returned when the IHI status is expired |
Retired | 01614 | Error: The IHI Number will not be returned when the IHI status is Retired |
Resolved | 01606 | Information: This IHI record is part of a resolved duplicate group resolved to IHI Number <IHI Number>. |
Resolved | 01611 | Information: This IHI record is a duplicate IHI record that has been resolved to IHI number <IHI Number>. |
Resolved | 01612 | Information: This IHI record is provisional IHI record that has been resolved to IHI Number <IHI Number>. |
To find out the service messages, see the code below.
// searchIHIResponse is available class in installed NEHTA library.
searchIHIResponse ihiResponse = client.BasicMedicareSearch(request);
var serviceMessages = ihiResponse.searchIHIResult.serviceMessages
Actual test case implementation;
private void SavePatientIHIDetails_HI_010_005820(Patient patient)
{
// HIHelperService is service class and contains HI Service related utility methods, e.g. IHI Lookup, IHI Validate, etc.
HIResponse ihiResponseObj = HIHelperService.SearchBy(patient.GivenName, patient.FamilyName, patient.DOB, patient.Gender, patient.MedicareNumber, patient.IHINumber, patient.DVA);
// IHI is not null or empty with IHI status "Active" or "Deceased"
if (!string.IsNullOrEmpty(ihiResponseObj.IHINumber))
{
patient.IHINumber = ihiResponseObj.IHINumber;
patient.IHIStatus = ihiResponseObj.IHIStatus;
patient.IHIRecordStatus = ihiResponseObj.IHIRecordStatus;
patient.IHIUpdatedDate = DateTime.Now;
}
else
{
// service messages are retrieved as.
// ihiResponse = client.BasicMedicareSearch(request); where client is ConsumerSearchIHIClient. Please see IHI lookup example.
// ihiResponse.searchIHIResult.serviceMessages.serviceMessage
// Please check the IHI look up tutorial to find more.
// In this demo, HIHelperService is a local service class that does all the job under the hood, and put the HI response with the service messages into local HIResponse object
var serviceMessage = ihiResponseObj.ServiceMessages.serviceMessage[0];
// When IHI is retired, expired or resolved.
switch (serviceMessage.code)
{
// Error Code 01613 = "Expired"
case "01613":
patient.IHIStatus = "Expired";
patient.IHIError = serviceMessage.reason;
break;
// Error Code 01614 = "Retired"
case "01614":
patient.IHIStatus = "Retired";
patient.IHIError = serviceMessage.reason;
break;
// Information Code: 01606, 01611, and 01612 when IHI is resolved to new <IHI Number>
case "01606":
case "01611":
case "01612":
// Get the new IHI number from the HI Service response.
// Get the old IHI number (your local CIS database) and //save // into patient's old ihi.
// Store the service messages.
break;
}
_patientService.SavePatient(patient);
}
}
Test Case ID | HI_010_005839 |
---|---|
Test Objective | The software shall raise an alert whenever an IHI is assigned to a patient record and the same IHI has already been assigned to one or more other records of patients in the local system. |
How to evaluate | Perform a patient registration where the software processes an IHI assignment with the same IHI already assigned to one or more other records of patients in the local system: a. Verify that software raises an alert whenever an IHI is assigned to a patient record and the same IHI has already been assigned to one or more other records of patients in the local system. |
Code Example
The following code example demonstrates how to raise an error/alert when the same IHI number is assigned to more than one patient record. For the purpose of simplicity, assume we have a method in our local system to search for a patient and it throws an exception if the IHI is already linked with another patient. Your software would likely prompt the user that they should merge records or conduct some other activity.
public void SaveOrUpdatePatient(Patient patient)
{
try
{
searchIHI request = new searchIHI();
request.givenName = patient.GivenName;
request.familyName = patient.FamilyName;
request.sex = patient.SexType;
request.dateOfBirth = patient.DOB;
request.medicareCardNumber= patient.MedicareNumber
// Invokes a basic search
searchIHIResponse ihiResponse = client.BasicSearch(request);
// All details must be saved when saving IHI number.
patient.IHINumber = ihiResponse.searchIHIResult.ihiNumber;
patient.IHINumberStatus = ihiResponse.searchIHIResult.ihiStatus;
patient.UpdatedAt = DateTime.Now;
patient.IHIRecordStatus = ihiResponse.searchIHIResult.ihiRecordStatus;
// Verify IHI number is not already attached with linked with another patient.
// This code throws some kind data exception if IHI number is found with existing patient in the local system.
VerifyIHINumberInExistingPatientRecord(patient);
patientRepository.SaveOrUpdatePatient(patient);
}
catch (IncorrectDataException de)
{
MessageBox("This IHI number is already attached with existing patient.");
return;
}
}
Test Case ID | HI_010_005843 |
---|---|
Test Objective | The Software shall have the capability to display the IHI number assigned to a patient, the IHI number status and the IHI record status. |
How to evaluate | Associate an IHI number to a newly-created patient health record: a. Verify that software displays the IHI number assigned to a patient. b. Verify that software displays the IHI number status. c. Verify that software displays the IHI record status. |
The following screenshot shows the IHI details linked with a newly created patient.
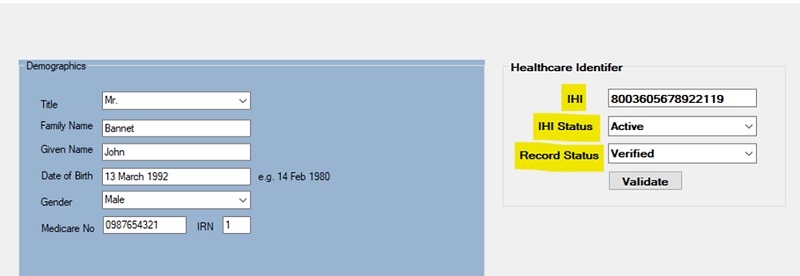
Test Case ID | HI_010_005873 |
---|---|
Test Objective | The software shall create an error log for all error messages received from the HI Service including those that are resolved automatically. The log shall include the error date and time, in hours and minutes unless the system is capable of more precision, the error number, the error message and message ID reported by the HI Service. |
How to evaluate | Perform a patient registration operation which includes an error being returned from the HI Service. View the log entries in the local software. a. Verify that the software created log entries for all error messages received from the HI Service including those that are resolved automatically. b. Verify that the log entries include the error date and time, in hours and minutes unless the system is capable of more precision c. Verify that the log entries include the error number reported by the HI Service. d. Verify that the log entries include the error message reported by the HI Service. e. Verify that the log entries include the message ID reported by the HI Service. |
Code example
The following code example demonstrates the error messages returned from the HI Service and can be saved either in the file system or database using the logging service of your software.
Let’s take an assumption the web service call throws a fault exception in case of an incorrect request or service message if record is not matched. The following code handles both. It is highly important to interrogate "serviceMessage" to ensure the request was successful.
// Set up the request IHI
searchIHI request = new searchIHI();
request.medicareCardNumber = "495015297";
request.medicareIRN = "1";
request.dateOfBirth = DateTime.Parse("22 Aug 1980");
request.givenName = "John";
request.familyName = "Kelleher";
request.sex = SexType.M;
try
{
// Invokes a basic search
searchIHIResponse ihiResponse = client.BasicMedicareSearch(request);
// Error logging from the returned response.
if (ihiResponse.searchIHIResult.serviceMessages != null &&
ihiResponse.searchIHIResult.serviceMessages.serviceMessage.Length>0)
{
// Log details e.g.
InfoLog info = new infoLog();
info.ErrorMessage = ihiResponse.searchIHIResult
.serviceMessages.serviceMessage[0].reason;
info.ErrorId = client.SoapMessages.SoapRequestMessageId;
info.ErrorDateTime = DateTime.Now;
errorDetails.ErrorNumber = client.SoapMessages.SoapResponseMessageId;
info
// Log error in Database.
DbLogger.LogInfo(errorDetails);
}
return ihiResponse.searchIHIResult.ihiRecordStatus.ToString();
}
catch (FaultException fex)
{
string returnError = "";
MessageFault fault = fex.CreateMessageFault();
if (fault.HasDetail)
{
ServiceMessagesType error = fault.GetDetail<ServiceMessagesType>();
// Look at error details in here
if (error.serviceMessage.Length > 0)
returnError = error.serviceMessage[0].code + ": "
+ error.serviceMessage[0].reason;
}
// If an error is encountered, client.LastSoapResponse often provides a more
// detailed description of the error.
string soapResponse = client.SoapMessages.SoapResponse;
string requestMessageId = client.SoapMessages.SoapRequestMessageId;
string responseMessageId = client.SoapMessages.SoapResponseMessageId;
ErrorLog errorDetails = new ErrorLog();
errorDetails.ErrorMessage = returnError;
errorDetails.ErrorId = requestMessageId;
errorDetails.ErrorNumber = responseMessageId;
errorDetails.ErrorDateTime = DateTime.Now;
// Log error in File System
FileLogger.LogError(errorDetails);
// Log error in Database.
DbLogger.LogError(errorDetails);
}
Test Case ID | HI_010_005875 |
---|---|
Test Objective | If an IHI with a supported record status is returned from the HI Service for a patient, the software shall have the capacity to assign that IHI to the patient's record and raise an alert if the search criteria used matches another patient's demographic data from the same registration source. If an alert is raised, the system shall either discard the IHI or store it against the target patient record and flag the records as potentially conflicting. |
How to evaluate | When saving new patient record, verify: a. if patient record with same IHI number already exist and raise alert. b. If software operator still wants to save patient, flag the patient record with conflicted. |
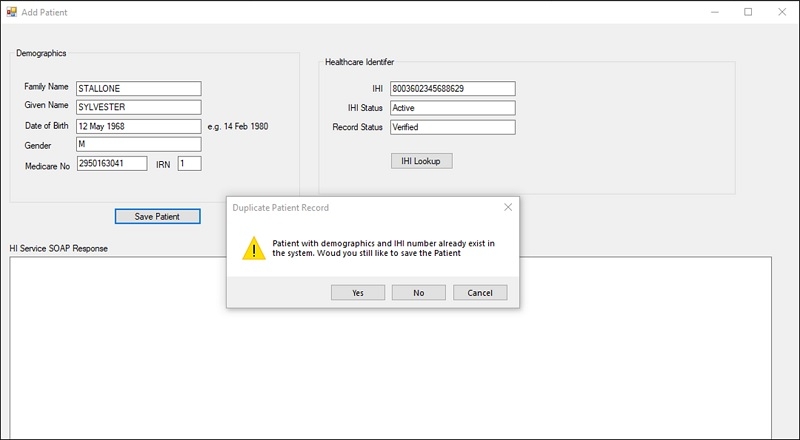
The following code example demonstrates how to warn the user (with an alert) when the patient is saved with a verified IHI number that has the same demographic data as another patient already present in the local software.
private void btnSavePatient_Click(object sender, EventArgs e)
{
Patient patient = new Patient();
patient.FamilyName = txtFamilyName.Text;
patient.GivenName = txtGivenName.Text;
patient.DOB = txtDob.Text;
patient.Gender = txtGender.Text;
patient.MedicareNo = txtMedicareNo.Text;
patient.IHINumber = txtIHINumber.Text;
patient.IHIStatus = txtIHIStatus.Text;
Patient patientRecordFound = _patientRepo
.GetPatientByDemographicMatchingIHINumber(patient);
if(patientRecordFound != null)
{
DialogResult dialogResult = MessageBox.Show("A patient with the same demographics and IHI already exists in the system. Would you still like to save the patient?", "Duplicate Patient Record", MessageBoxButtons.YesNoCancel, MessageBoxIcon.Warning);
if (dialogResult == DialogResult.Yes)
{
// Flag the both patients as conflicted.
bool newPatientSaved = _patientRepo.SavePatientWithFlagConflicted(patient);
bool newPatientSaved = _patientRepo.SavePatientWithFlagConflicted(patientRecordFound);
}
else if (dialogResult == DialogResult.No)
{
//do something else
}
}
Test Case ID | HI_010_008028 |
---|---|
Test Objective | The software shall have the ability to record an audit trail of all healthcare identifiers disclosed by the HI Service regardless of type. The audit trail shall be retrievable. The audit trail shall record at least the following items:
|
How to evaluate | Perform a patient registration operation and verify all above information is saved in patient record for audit trail, software shall have ability to view the Audit trail. |
This example demonstrates that each time a new patient is saved, all fields mentioned in the test objective must be saved for the audit trail.
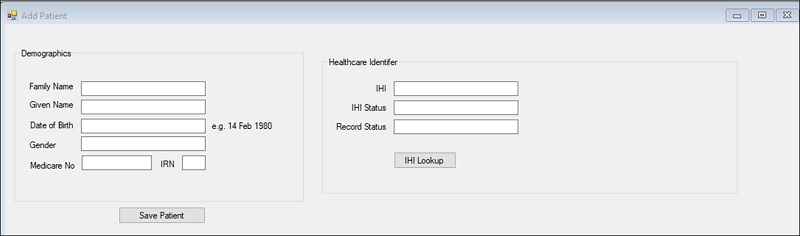
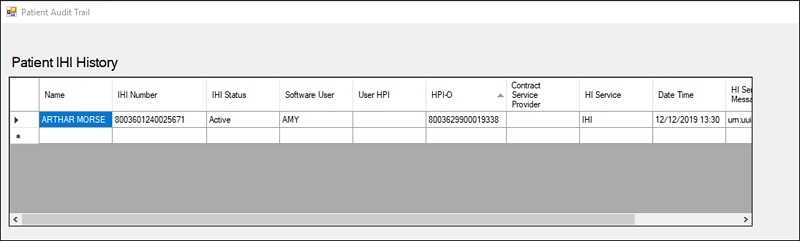
Code example
private void btnSavePatient_Click(object sender, EventArgs e)
{
Patient patient = new Patient();
patient.FamilyName = txtFamilyName.Text;
patient.GivenName = txtGivenName.Text;
patient.DOB = txtDob.Text;
patient.Gender = txtGender.Text;
patient.MedicareNo = txtMedicareNo.Text;
patient.IHINumber = txtIHINumber.Text;
patient.IHIStatus = txtIHIStatus.Text;
PatientIHIHistory patientIHIData = new PatientIHIHistory();
patientIHIData.LocalID = "Local Software Identifier";
patientIHIData.Name = patient.FamilyName + patient.GivenName;
patientIHIData.IHINumber = patient.IHINumber;
patientIHIData.IHIStatus = patient.IHIStatus;
patientIHIData.User = "Local Software User";
patientIHIData.UserHPI = "HPI if available";
patientIHIData.HIService = "e.g. IHI Lookup";
patientIHIData.HPIO = "HPI-O";
patientIHIData.HIService_Operation = HI_WebService_NAME;
patientIHIData.HIService_Version = HI_WebService_Version;
patientIHIData.HIServiceMessageID = "HI Message ID";
patient.PatientIHIHistory.Add(patientIHIData);
_patientRepo.SavePatient(patient);
}
Clarification
Web Service Name and version can be found in TECH.SIS HI service guides from the Services Australia and can be saved in your application configuration file (i.e. App.Config) or database.
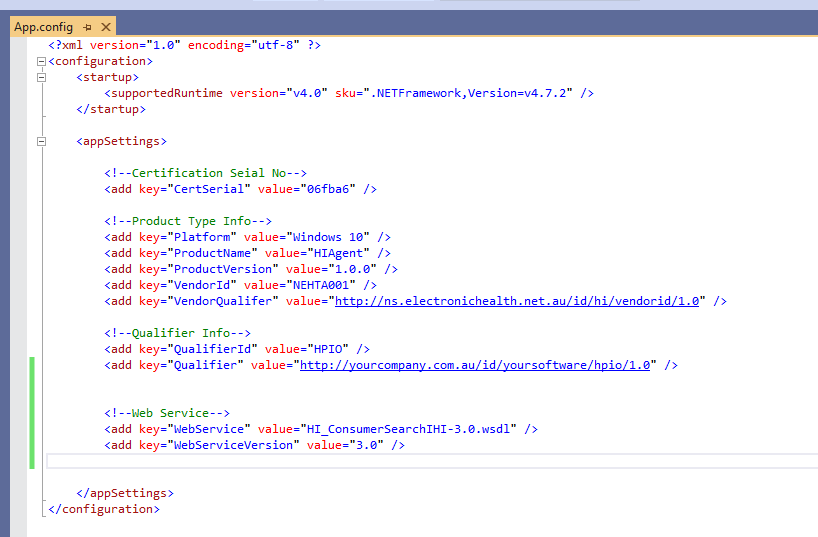
private readonly static string HI_WebService_NAME = ConfigurationManager
.AppSettings.Get("WebServiceName");
private readonly static string HI_WebService_Version = ConfigurationManager
.AppSettings.Get("WebServiceVersion");
Clarification
HI Service Message ID can be found in the SOAP Response Message. Please see the below code. When the client makes a request, it returns the SOAP response.
Full SOAP Web service response
<?xml version="1.0" encoding="UTF-8"?>
<soap12:Envelope xmlns:soap12="http://www.w3.org/2003/05/soap-envelope"><soap12:Header><wsa:Action xmlns:wsa="http://www.w3.org/2005/08/addressing">http://ns.electronichealth.net.au/hi/svc/ConsumerSearchIHI/3.0/ConsumerSearchIHIPortType/searchIHIResponse</wsa:Action><wsa:MessageID xmlns:wsa="http://www.w3.org/2005/08/addressing">urn:uuid:fd8b53c3-80fa-49c6-b99b-e891d347bcc0</wsa:MessageID><wsa:RelatesTo xmlns:wsa="http://www.w3.org/2005/08/addressing" RelationshipType="http://www.w3.org/2005/08/addressing/reply">urn:uuid:43098eb6-9696-4b61-8e6c-1d9cfed5b6f3</wsa:RelatesTo><wsa:To xmlns:wsa="http://www.w3.org/2005/08/addressing">http://www.w3.org/2005/08/addressing/anonymous</wsa:To><audit:product xmlns:audit="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0"><audit:vendor><qid:qualifier xmlns:qid="http://ns.electronichealth.net.au/hi/xsd/common/QualifiedIdentifier/3.0">http://ns.medicareaustralia.gov.au/distinguishedname/1.0</qid:qualifier><qid:id xmlns:qid="http://ns.electronichealth.net.au/hi/xsd/common/QualifiedIdentifier/3.0">CN=Medicare Australia :6278034019,O=Medicare Australia,L=TUGGERANONG,ST=ACT,C=AU</qid:id></audit:vendor><audit:productName>HI</audit:productName><audit:productVersion>1.0</audit:productVersion><audit:platform>MedicareAustralia</audit:platform></audit:product><audit:signature xmlns:audit="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0" xmlns:qid="http://ns.electronichealth.net.au/hi/xsd/common/QualifiedIdentifier/3.0"><Signature xmlns="http://www.w3.org/2000/09/xmldsig#" xmlns:wsse="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd"><SignedInfo><CanonicalizationMethod Algorithm="http://www.w3.org/2001/10/xml-exc-c14n#"/><SignatureMethod Algorithm="http://www.w3.org/2000/09/xmldsig#rsa-sha1"/><Reference URI="#Id-eb7cacc2-8f31-4ea2-ae7d-e891d347a103"><Transforms><Transform Algorithm="http://www.w3.org/2001/10/xml-exc-c14n#"/></Transforms><DigestMethod Algorithm="http://www.w3.org/2000/09/xmldsig#sha1"/><DigestValue>D9IEYD8kyNn6/onXUr8opgKk3Y4=</DigestValue></Reference></SignedInfo><SignatureValue>EEpKm9Wt2EXTUEsoiSiGZCFiu4y5aKFaUQnf9VGE0iPNrqeCtSPn1zBTeQNtT/NjL2hDgFfkof7Yl/9PPJcCSE7U8h6tBg7MJgoparjyPqtbsdyNg3lGvOVGWduvZFWQQZ2o+D1YydVEG335WJUQCJMSvnFDNw0Rt/eUUSoYqIx4OI2/KTNZ4izqTtEo8JdF25BIDEDKT+mGcXJlqQ3NYXmBboB9f8EFgh2WveO4kQYCv6KBsA69pwgRCG1t+Wv8x45b0WSYBCp4gBW5XSOU8kQNX67kJ6EmKJnzvIozqeWEdSM5bmt2+t8/Hg9FLuzaekly0ReD+4OEGWyPKivnZg==</SignatureValue><ds:KeyInfo xmlns="" xmlns:ds="http://www.w3.org/2000/09/xmldsig#"><ds:X509Data><ds:X509Certificate>MIIFyTCCBLGgAwIBAgIDBsExMA0GCSqGSIb3DQEBBQUAMH8xCzAJBgNVBAYTAkFVMQwwCgYDVQQKEwNHT1YxGzAZBgNVBAsTEk1lZGljYXJlIEF1c3RyYWxpYTFFMEMGA1UEAxM8VGVzdCBNZWRpY2FyZSBBdXN0cmFsaWEgT3JnYW5pc2F0aW9uIENlcnRpZmljYXRpb24gQXV0aG9yaXR5MB4XDTE4MDcxODA2MTkwNFoXDTIyMDMwNTAwNTQxMFowfDELMAkGA1UEBhMCQVUxDDAKBgNVBAgTA0FDVDEUMBIGA1UEBxMLVFVHR0VSQU5PTkcxGzAZBgNVBAoTEk1lZGljYXJlIEF1c3RyYWxpYTEsMCoGA1UEAxMjVGVzdCBNZWRpY2FyZSBBdXN0cmFsaWEgOjk0MjE4NzAzNTQwggEiMA0GCSqGSIb3DQEBAQUAA4IBDwAwggEKAoIBAQCph8PA14r2K0TMy9efrpT9adscGcj374/Dzvu2H/csqILusHjsB/d7TnVVV7D6StWCxYn/kf2wuZMFdkrEdCjsSHfbTHPGgtFPcxunorYTPHEtSe5p0dp/IZML7tUCiK48ywOTipDZ/Xiyxokt2bVjnq5AAe1WWou5ZJYJ+E7D7v6aRD/SwDQXoq/D/iq9Prg9lYzeXFnKgCfsLuKQiEweU6GVSy/S4uT6i0U91k605vbIV5HtSIDuzLikZaYD6uUDB/pK8l2h8CpkxlKvtLYZxOskKfzopgrWse/ZC73e+1CBTd4FjuGXU6xm8suybxD1gOpqogY4gXCyJHh11uNrAgMBAAGjggJPMIICSzAMBgNVHRMBAf8EAjAAMB8GA1UdEQQYMBaBFGVidXMudGVzdEBoaWMuZ292LmF1ME8GCCsGAQUFBwEBBEMwQTA/BggrBgEFBQcwAYYzaHR0cDovL29jc3AuY2VydGlmaWNhdGVzLWF1c3RyYWxpYS5jb20uYXUvbWFvY2EucGt4MBkGCSoko5CVFwHOGQQMFgo5NDIxODcwMzU0MIIBIQYDVR0gBIIBGDCCARQwggEQBgoqJNL+gHcBBAIBMIIBADCBywYIKwYBBQUHAgIwgb4agbtDZXJ0aWZpY2F0ZXMgaXNzdWVkIHVuZGVyIHRoaXMgQ1AgbXVzdCBvbmx5IGJlIHJlbGllZCBvbiBieSBlbnRpdGllcyB3aXRoaW4gdGhlIENvbW11bml0eSBvZiBJbnRlcmVzdCwgdW5sZXNzIG90aGVyd2lzZSBhZ3JlZWQsIGFuZCBub3QgZm9yIHB1cnBvc2VzIG90aGVyIHRoYW4gdGhvc2UgcGVybWl0dGVkIGJ5IHRoaXMgQ1AuMDAGCCsGAQUFBwIBFiRodHRwOi8vd3d3Lm1lZGljYXJlYXVzdHJhbGlhLmdvdi5hdS8wDgYDVR0PAQH/BAQDAgeAMBMGA1UdIwQMMAqACECvQwoiM+vJMFEGA1UdHwRKMEgwRqBEoEKGQGh0dHA6Ly9tYXRlc3QuY2VydGlmaWNhdGVzLWF1c3RyYWxpYS5jb20uYXUvVGVzdE1BT0NBL2xhdGVzdC5jcmwwEQYDVR0OBAoECEl266/wkvKQMA0GCSqGSIb3DQEBBQUAA4IBAQBJhbptvpSumSoipJhbQYDgGaHjb684BGh1ozzyNM8wDorxaxmEAVGZxfFf2nkNhKcLL4DTbcz2uvAiLfS4LsHaJiU7DpgdCTkQbcaXszTMPt3pKZ9xV/GqQqhbLa/e2/HzxlcLIbx2ELBn6ywVW9W2PkGQOLJdiIa8upe1KoSegzid5qjaIoZYdig4HC6HYTjkvgYR4xNT4AGQbUBs13QMnxQuvT67jNp8vFMJNYkydo7ZW/WEIVnBo+rneKisml+Bg2980kxF8bhyraTgHoQfS3DovBEvQU4fx/AhC12kb+uy3Ne0ghYcycmfl4rsdOIydFX/VJwcg6rCzoXzZnoH</ds:X509Certificate></ds:X509Data></ds:KeyInfo></Signature></audit:signature></soap12:Header><soap12:Body xml:id="Id-eb7cacc2-8f31-4ea2-ae7d-e891d347a103"><consWSDL:searchIHIResponse xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:consWSDL="http://ns.electronichealth.net.au/hi/svc/ConsumerSearchIHI/3.0"><out4:searchIHIResult xmlns:out4="http://ns.electronichealth.net.au/hi/xsd/consumermessages/SearchIHI/3.0"><out2:ihiNumber xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">http://ns.electronichealth.net.au/id/hi/ihi/1.0/8003602345688629</out2:ihiNumber><out2:medicareCardNumber xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">2950163041</out2:medicareCardNumber><out2:ihiRecordStatus xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">Verified</out2:ihiRecordStatus><out2:ihiStatus xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">Active</out2:ihiStatus><out:givenName xmlns:out="http://ns.electronichealth.net.au/hi/xsd/common/IndividualNameCore/3.0">SYLVESTER</out:givenName><out:familyName xmlns:out="http://ns.electronichealth.net.au/hi/xsd/common/IndividualNameCore/3.0">STALLONE</out:familyName><out5:dateOfBirth xmlns:out5="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0">1968-05-12</out5:dateOfBirth><out5:sex xmlns:out5="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0">M</out5:sex></out4:searchIHIResult></consWSDL:searchIHIResponse></soap12:Body></soap12:Envelope>
Important!
Please review all other Test Cases for UC.010. This guide has not completely described all Test Cases:
Healthcare Identifiers Service - Support Documents - Conformance Test Specification v3.4
Use Case 015 (UC.015) – Update the Patient Record
This guide will show you how to apply Test Cases for UC.015 when updating a patient in your system. To see to all test cases related to update patient, please refer to:
Healthcare Identifiers Service - Support Documents - Conformance Test Specification v3.4
The following is an example of a Test Case which has the same requirement covered by a Test Case for UC.010. If you have addressed that Test Case, no action should be needed.
Use Case Name | Update patient health record |
Purpose | Create a new patient health record. |
Outline | To ensure that we identify this patient in a manner consistent with the HI Service and to have the most up to date information available for the patient. Compare the information received about the patient to the details within the software, and update the patient’s record with the new details if they have changed. Where appropriate, the IHI details are verified or updated within the HI Service. If the new details about the patient include a date of death, the HI Service is notified of the patient’s date of death. |
Test Case ID | HI_015_005801 |
---|---|
Test Objective | If the software supports the capture of IHIs via manual or OCR input then the software shall ensure that whenever an IHI is captured using manual or OCR input all sixteen digits are included, the identifier is stored as 16 continuous digits (no spaces) and the identifier is validated using the Luhn check digit algorithm (see appendix B). If the IHI does not include sixteen continuous digits or fails the Luhn check digit algorithm the IHI shall not be stored and an operator will be alerted. |
How to evaluate | See the Test Specification Excel file for this section |
Code Example
This code demonstrates the 16 number digit check using C# regular expression.
public bool VerifyIHINumber(string ihiNumber)
{
var match = Regex.Match(ihiNumber, @"800360\d{10}");
return match.Success;
}
Test Case ID | HI_015_005802 |
---|---|
Test Objective | If the software permits the capture of a patient demographic record, the software should permit the manual entry of an IHI. |
How to evaluate | When updating the patient health record, verify: a. Software allows manual entry of IHI number b. Verify that IHI is entered and is displayed along with the patient health record. |
Demo screenshot shows when a patient is being updated, user can enter IHI number manually and revalidate.
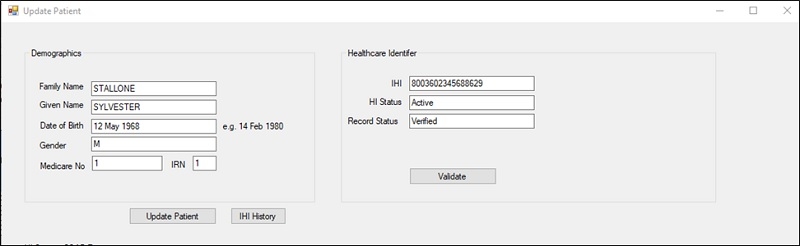
Test Case ID | HI_015_016813 |
---|---|
Test Objective | When a verified IHI is validated and the HI Service returns a ‘resolved’ information message and a different IHI number, the software shall not store that new IHI unless it can also be validated with the existing patient demographics in the local system. If the new IHI cannot be validated with the local patient demographic data, then an alert shall be raised so an operator can determine what action should be taken. The new IHI number, IHI status and IHI record status shall be stored in the patient record if the IHI number can be validated using local patient demographic data. The old IHI shall be moved to the patient record history with a resolved status regardless of the validity of the new IHI. |
Explanation | This test case tells that when a patient’s IHI is revalidated (e.g. required by My Health Record) with the HI Service, the IHI may be found as “resolved”. If this situation occurs, the “resolved” status is not returned in the IHI Status field, instead it comes as part of the service message from the web service. The resolved-to IHI (new) IHI will be returned, together with the associated IHI Status and Record Status. This resolved-to IHI needs to be validated with demographics saved in your local software before it can be saved, and the resolved (old) IHI is moved into patient historical data. Please see the code to understand the logic. |
How to evaluate | Update a patient record with verified IHI, for which HI Service returns resolved information and a different IHI. a. Verify that the system revalidates the returned IHI with the local demographic data. b. If the returned IHI can be validated successfully, ensure the old IHI is moved to the patient record history and the returned IHI is stored in the patient record c. Verify that if the new IHI cannot be validated with the local patient demographic data then an alert shall be raised so an operator can determine what action should be taken d. Verify the old IHI is moved to the patient record history with a resolved status regardless of the validity of the new IHI. |
Important!
“Resolved” returns as a web response service message with code “01611”. This means the IHI has been resolved to a new IHI.
// ------------------------------------------------------------------------------
// Client instantiation and invocation
// ------------------------------------------------------------------------------
// Instantiate the client
ConsumerSearchIHIClient client = new ConsumerSearchIHIClient(
new Uri("https://www5.medicareaustralia.gov.au/cert/soap/services/"),
product,
user,
hpio,
signingCert,
tlsCert);
// Set up the request IHI
searchIHI request = new searchIHI();
request.ihiNumber = "http://ns.electronichealth.net.au/id/hi/ihi/1.0/" + patient.IHINumber;
request.dateOfBirth = DateTime.Parse(patient.DOB);
//request.givenName = "Emily";
request.familyName = patient.FamilyName;
request.sex = SexType.M;
try
{
// Invokes a basic search
searchIHIResponse ihiResponse = client.BasicSearch(request);
string ihiNumber = ihiResponse.searchIHIResult.ihiNumber;
// Check IHI is resolved, and new IHI has been returned.
if (ihiResponse.searchIHIResult.serviceMessages.serviceMessage[0].code == "01611")
{
// verify returned demographics with local data.
var returndPatientData = new Patient()
{
FamilyName = ihiResponse.searchIHIResult.familyName,
GivenName = ihiResponse.searchIHIResult.givenName,
DOB = ihiResponse.searchIHIResult.dateOfBirth.ToString()
// Other patient info
};
if (ValidatedWithLocalData(patient, returndPatientData))
{
// Move old IHI into Patient record history with resolved status.
// Save Patient data with new returned IHI
}
else
{
// If new IHI cannot be validated with the local patient data, then alert user.
DialogResult dialogResult = MessageBox.Show("HI returns with resolved status, with new IHI " + ihiNumber + ", but demographics cannot be validated with local data.", "IHI Resolved", MessageBoxButtons.YesNoCancel,MessageBoxIcon.Exclamation);
// Give option to operator and determine what action should be taken.
}
}
}
catch (FaultException fex)
{
string returnError = "";
MessageFault fault = fex.CreateMessageFault();
if (fault.HasDetail)
{
ServiceMessagesType error = fault.GetDetail<ServiceMessagesType>();
//error.serviceMessage.
// Look at error details in here
if (error.serviceMessage.Length > 0)
returnError = error.serviceMessage[0].code + ": " + error.serviceMessage[0].reason;
}
// If an error is encountered, client.LastSoapResponse often provides a more
// detailed description of the error.
string soapResponse = client.SoapMessages.SoapResponse;
txtSoapResponse.Text = soapResponse;
}
catch (Exception ex)
{
// handle any errors.
}
The following is the SOAP response body in which the HI Service returned “01611” as the service message code with the new IHI number.
<soap12:Body xml:id="Id-c075083e-280b-40ec-acec-e891d347561f">
<consWSDL:searchIHIResponse
xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:consWSDL="http://ns.electronichealth.net.au/hi/svc/ConsumerSearchIHI/3.0">
<out4:searchIHIResult
xmlns:out4="http://ns.electronichealth.net.au/hi/xsd/consumermessages/SearchIHI/3.0">
<out2:ihiNumber
xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">http://ns.electronichealth.net.au/id/hi/ihi/1.0/8003601240025671
</out2:ihiNumber>
<out2:ihiRecordStatus
xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">Verified
</out2:ihiRecordStatus>
<out2:ihiStatus
xmlns:out2="http://ns.electronichealth.net.au/hi/xsd/consumercore/ConsumerCoreElements/3.0">Active
</out2:ihiStatus>
<out:givenName
xmlns:out="http://ns.electronichealth.net.au/hi/xsd/common/IndividualNameCore/3.0">ARTHUR
</out:givenName>
<out:familyName
xmlns:out="http://ns.electronichealth.net.au/hi/xsd/common/IndividualNameCore/3.0">MORSE
</out:familyName>
<out5:dateOfBirth
xmlns:out5="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0">2000-12-12
</out5:dateOfBirth>
<out5:sex
xmlns:out5="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0">M
</out5:sex>
<out5:serviceMessages
xmlns:out5="http://ns.electronichealth.net.au/hi/xsd/common/CommonCoreElements/3.0">
<out5:highestSeverity>Informational</out5:highestSeverity>
<out5:serviceMessage>
<out5:code>01611</out5:code>
<out5:severity>Informational</out5:severity>
<out5:reason>(INFORMATION) This IHI record is a duplicate IHI record that has been resolved to IHI number 8003601240025671.</out5:reason>
</out5:serviceMessage>
</out5:serviceMessages>
</out4:searchIHIResult>
</consWSDL:searchIHIResponse>
</soap12:Body>
Test Case ID | HI_015_016815 |
---|---|
Test Objective | When an active and verified IHI is validated and the HI Service returns the same verified IHI but with a different number status, the software shall either store the new status in the patient record or raise a warning or alert according to the following table. Active: No change |
How to evaluate | Update a patient record with an active and verified IHI that is known to the local system so that it will return a deceased status: a. Ensure the software raises a warning Update a patient record with an active and verified IHI that is known to the local system so that it will return a retired status: b. Ensure the software stores the new status and raises a warning * Expired IHI status cannot be tested but software should handle the condition of an expired status being returned for a verified IHI by raising an alert. This can be confirmed via code inspection, database manipulation or test harness that supports this transition. |
This code example demonstrates how to handle the IHI status responses returned by the HI Service. When IHI lookup request is made for validation. It raises a fault exception when the status is “Retired”
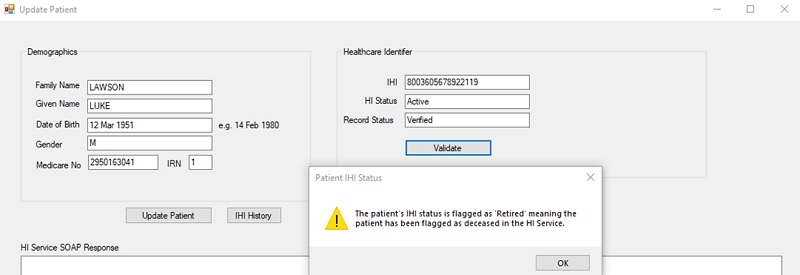
try
{
// Invokes a basic search
searchIHIResponse ihiResponse = client.BasicSearch(request);
txtIHINumber.Text = ihiResponse.searchIHIResult.ihiNumber;
txtIHIStatus.Text = ihiResponse.searchIHIResult.ihiStatus.ToString();
txtRecordStatus.Text = ihiResponse.searchIHIResult.ihiRecordStatus.ToString();
switch (ihiResponse.searchIHIResult.ihiStatus.ToString())
{
case "Deceased":
MessageBox.Show("Deceased", "", MessageBoxButtons.OK, MessageBoxIcon.Information);
break;
case "Expired":
MessageBox.Show("Expired", "", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
break;
default:
MessageBox.Show("Nothing has been changed.");
break;
}
}
catch (FaultException fex)
{
string returnError = "";
MessageFault fault = fex.CreateMessageFault();
if (fault.HasDetail)
{
ServiceMessagesType error = fault.GetDetail<ServiceMessagesType>();
//error.serviceMessage.
// Look at error details in here
if (error.serviceMessage.Length > 0)
returnError = error.serviceMessage[0].code + ": " + error.serviceMessage[0].reason;
}
if (returnError.Contains("Retired"))
{
DialogResult dialogResult = MessageBox.Show("The patient’s IHI status is flagged as ‘Retired’ meaning the patient has been flagged as deceased in the HI Service.", "Patient IHI Status", MessageBoxButtons.OK, MessageBoxIcon.Warning);
// Save new status.
}
txtError.Text = returnError;
// If an error is encountered, client.LastSoapResponse often provides a more
// detailed description of the error.
string soapResponse = client.SoapMessages.SoapResponse;
txtSoapResponse.Text = soapResponse;
}
Conclusion
You should now be comfortable with the web services, Use Cases, and Test Cases for IHI Lookups, which are used to uniquely identify patients. This functionality is a requirement for many aspects of Digital Health such as Secure Messaging, Electronic Prescribing and the My Health Record system.
The next guide will walk through verifying healthcare professionals in the system by validating their HPI-Is.
View All | Back | Next: Search for HPI-I - HI Service Developer Guide 3