Reading & Development: 2 hours
Quick Intro
It is highly recommended that your software conduct HPI-I searches for users of your system. The primary reason for this is that most healthcare provider individuals do not know their HPI-I number however their AHPRA number is often known. The Agency has significant negative user feedback where products have not implemented HPI-I searches, specifically pointing towards the advantages of conducting HPI-I searches using AHPRA numbers where possible. HPI-I searches can be done in batch, or individually. We will look at individual searches in this guide. To do an HPI-I search you will send the provider’s information to the web service, and you will receive back an HPI-I or information as to why an HPI-I could not be returned. This guide is primarily addressing UC.131.
Important!
Ensure you have access to the HI Service licenced material and specifically the document for TECH.SIS.HI.31 which will describe the specifications of the SearchForProviderIndividual web service used in this guide. Page 7 provides a detailed description of the request and response parameters.
At the end of this walkthrough, you'll have a Windows form that conducts an HPI-I search using the registration id (AHPRA number) and family name values. Within your application this functionality would likely be combined into your existing user setup screen with HPI-Is searched when a new user is added, a user is updated, or as part of another workflow when there are users who do not have an HPI-I in the local system.
We have added a Search HPI-I button to our main form in the first screenshot below. In the 2nd screenshot you will see our fields for searching for the provider’s HPI-I. In your application this would likely be added to an existing user settings screen.
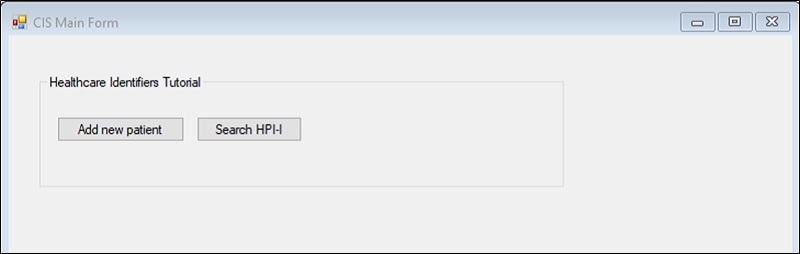
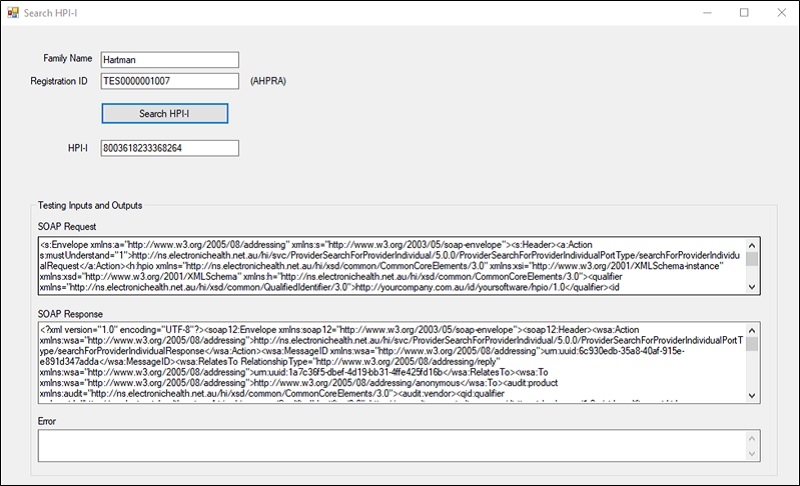
Step 1: Add a new Form
1. Add a new Windows Form called “Search HPI-I Form” in the existing project that we have created in the previous guide. If you have not followed previous guides, there are prerequisite steps you will have missed.
2. Add the following fields to the form and name them accordingly.
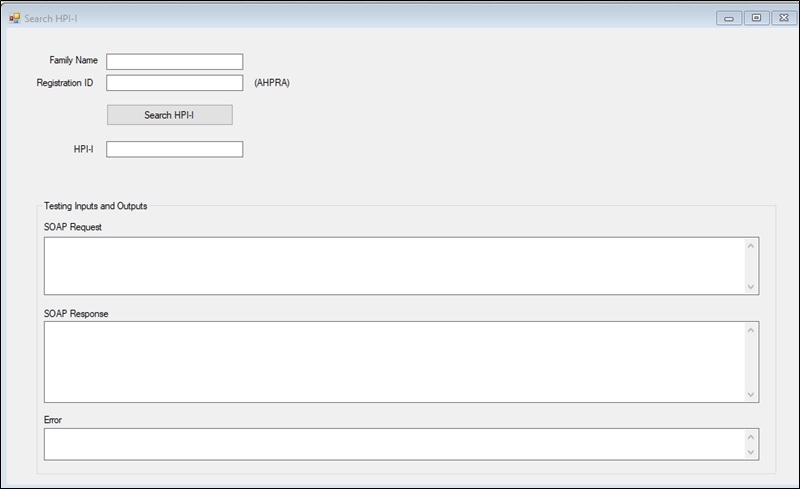
Step 2: Add code to conduct an HPI-I search
1. Right click on SeachHPI-IForm and click view code to open the class file. Add the following additional namespaces.
using System.ServiceModel;
using System.ServiceModel.Channels;
using System.Security.Cryptography.X509Certificates;
using Nehta.VendorLibrary.Common;
using nehta.mcaR50.ProviderSearchForProviderIndividual;
using System.Configuration;
using Nehta.VendorLibrary.HI;
2. Open App.Config from the Solution explorer and copy the SOAP client configuration inside the App.Config file, replacing the values which have been provided to you by Services Australia.
App.Config
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.7.2" />
</startup>
<appSettings>
<!--Certification Seial No-->
<add key="CertSerial" value="" />
<!--Product Type Info-->
<add key="Platform" value="Windows 10" />
<add key="ProductName" value=" " />
<add key="ProductVersion" value="" />
<add key="VendorId" value=" " />
<add key="VendorQualifer" value="http://ns.electronichealth.net.au/id/hi/vendorid/1.0" />
<!--Qualifier Info-->
<add key="QualifierId" value=" " />
<add key="Qualifier" value="http://yourcompany.com.au/id/yoursoftware/hpio/1.0" />
</appSettings>
</configuration>
3. Add the following code to the SearchHPI-IForm.cs class file to prepare the variables and assign the values from the App.Config file.
private readonly static string Certificate_Serial = ConfigurationManager
.AppSettings.Get("CertSerial");
private readonly static string Product_Platform = ConfigurationManager
.AppSettings.Get("Platform");
private readonly static string Product_Name = ConfigurationManager
.AppSettings.Get("ProductName");
private readonly static string Product_Version = ConfigurationManager
.AppSettings.Get("ProductVersion");
private readonly static string Vendor_Id = ConfigurationManager
.AppSettings.Get("VendorId");
private readonly static string Vendor_Qualifier = ConfigurationManager
.AppSettings.Get("VendorQualifer");
private readonly static string Qualifier_HPIO_Id = ConfigurationManager
.AppSettings.Get("QualifierId");
private readonly static string Qualifier_HPIO = ConfigurationManager.
AppSettings.Get("Qualifier");
4. The code should look like the screenshot below.
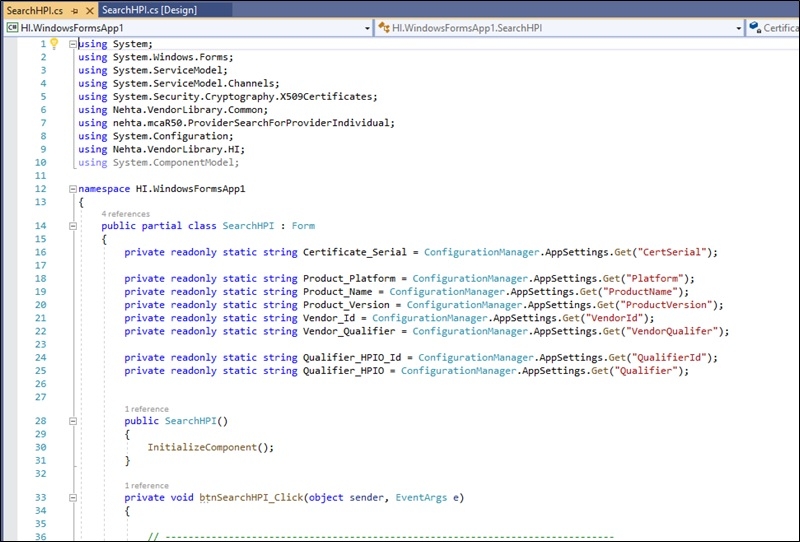
5. Double click on the Search HPI-I button to add the following code on the button click event.
private void btnSearchHPI-I_Click(object sender, EventArgs e)
{
// Obtain the certificate by serial number
X509Certificate2 tlsCert = X509CertificateUtil.GetCertificate(
Certificate_Serial,
X509FindType.FindBySerialNumber,
StoreName.My,
StoreLocation.CurrentUser,
true
);
// The same certificate is used for signing the request.
// This certificate will be different to TLS cert for some operations.
X509Certificate2 signingCert = tlsCert;
// Set up client product information (PCIN)
// Values below should be provided by Medicare
ProductType product = new ProductType()
{
platform = Product_Platform, // Can be any value
productName = Product_Name, // Provided by Medicare
productVersion = Product_Version, // Provided by Medicare
vendor = new QualifiedId()
{
id = Vendor_Id, // Provided by Medicare
qualifier = Vendor_Qualifier // Provided by Medicare
}
};
// Set up user identifier details
QualifiedId user = new QualifiedId()
{
// User ID internal to your system
id = "User Id",
// Eg: http://ns.yourcompany.com.au/id/yoursoftware/userid/1.0
qualifier = "http://<anything>/id/<anything>/userid/1.0"
};
// Set up organisation identifier details, only required for CSP software.
// If run as a CSP software, the certificate needs to be a CSP certificate
// Set up user identifier details
QualifiedId hpio = new QualifiedId()
{
id = Qualifier_HPIO_Id, // HPIO internal to your system
// Eg: http://ns.yourcompany.com.au/id/yoursoftware/userid/1.0
qualifier = Qualifier_HPIO
};
// ------------------------------------------------------------------------------
// Client instantiation and invocation
// ------------------------------------------------------------------------------
// Instantiate the client
var client = new ProviderSearchForProviderIndividualClient(
new Uri("https://www5.medicareaustralia.gov.au/cert/soap/services/"),
product,
user,
hpio,
signingCert,
tlsCert);
// Create the search request
var request = new searchForProviderIndividual()
{
familyName = txtFamilyName.Text,
registrationId = txtRegistrationId.Text,
};
try
{
// Invokes provider individual search.
var response = client.ProviderIndividualSearch(request);
var hpiSearchResult = response.searchForProviderIndividualResult;
txtSearchResult.Text = "";
if (hpiSearchResult.hpiiNumber != null)
{
txtSearchResult.Text = hpiSearchResult.hpiiNumber
.Substring(hpiSearchResult.hpiiNumber.Length - 16, 16);
}
else
{
txtError.Text = hpiSearchResult.serviceMessages
.serviceMessage[0].reason;
}
txtSOAPRequest.Text = client.SoapMessages.SoapRequest;
txtSOAPResponse.Text = client.SoapMessages.SoapResponse;
}
catch (FaultException fex)
{
string returnError = "";
MessageFault fault = fex.CreateMessageFault();
if (fault.HasDetail)
{
ServiceMessagesType error = fault.GetDetail<ServiceMessagesType>();
// Look at error details in here
if (error.serviceMessage.Length > 0)
returnError = error.serviceMessage[0].code + ": " + error
.serviceMessage[0].reason;
}
txtError.Text = returnError;
// If an error is encountered, client.LastSoapResponse often provides a more
// detailed description of the error.
string soapResponse = client.SoapMessages.SoapResponse;
txtSOAPResponse.Text = soapResponse;
}
catch (Exception ex)
{
txtSOAPResponse.Text = ex.StackTrace;
txtError.Text = ex.Message;
}
}
6. Add a button on your Main Form and following code to show the new “Search HPI form” Form.
private void button3_Click(object sender, EventArgs e)
{
SearchHPI-IForm hpi-iForm = new SearchHPI-IForm();
hpiForm.Show();
}
7. Run the application, open the Search HPI-I Form, and search for an HPI-I using the Registration Id and Family Name.
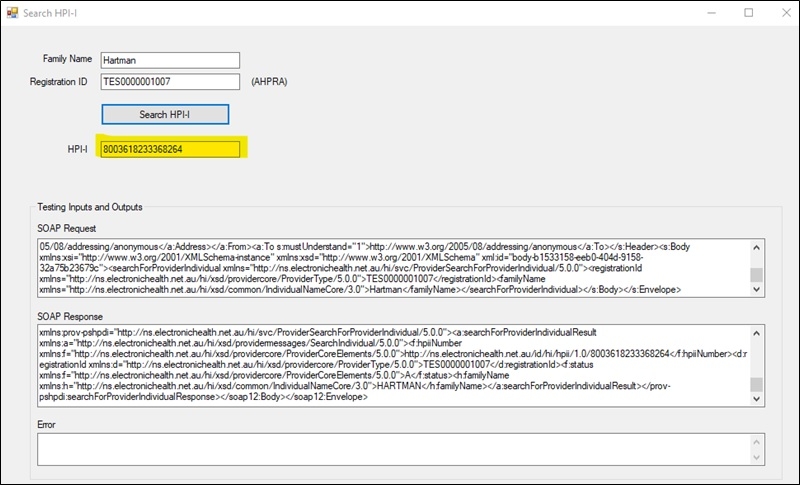
8. Conduct the search with both valid and invalid data to see the responses from the HI Service.
Step 3: Use Case 131 (UC.131)
We will now review several important & recommended test cases from UC.131. As usual it is important to note that the Test Cases identified below is not the complete list. View the complete list of Test Cases here: HI Service Use Cases.
Use Case Name | UC.131 |
Outline | To retrieve a Healthcare Provider Identifier Individual (HPI-I) record from the HI Service |
Test Case Coverage in this guide | HI_131_005873, HI_131_008028 |
Test Case ID | HI_131_005873 |
---|---|
Test Objective | The software shall create an error log for all error messages received from the HI Service including those that are resolved automatically. The log shall include the error date/time, in hours and minutes unless, the system is capable of more precision the error number, the error message and message ID reported by the HI Service. |
How to evaluate | The software shall create an error log for all error messages received from the HI Service including those that are resolved automatically. The log shall include the error date/time, in hours and minutes unless, the system is capable of more precision the error number, the error message and message ID reported by the HI Service. |
This test case is very similar to HI_010_005873 in UC.010 as it relates to logging your interactions with the HI Service. No further comment is necessary.
Test Case ID | HI_131_008028 |
---|---|
Test Objective | The software shall have the ability to record an audit trail of all healthcare identifiers disclosed by the HI Service regardless of type. The audit trail shall be retrievable. The audit trail shall record at least the following items: - The healthcare identifier disclosed by the HI Service - Any associated local record identifier(s) - Identifying information of the operator or responsible officer, including their HPI-I if applicable and known, that initiated access to the HI Service - The healthcare identifier (HPI-O) of the healthcare provider organisation that initiated the request to the HI Service - The CSP identifier of the contracted service provider (if applicable) - The HI Service operation (web service name) that disclosed the healthcare identifier - System date and time (time in hours and minutes unless the system is capable of more precision) - The HI Service messageID as documented by [TECH.SIS.HI.01] - The batch ID (if applicable) - The version of the HI Service web service |
How to evaluate | Verify the software have ability to record audit trail of all healthcare identifiers disclosed by the HI Service regardless of type that includes health identifier, associated local identifier, information of the operator or responsible officer, including their HPI-I if applicable, healthcare identifier (HPI-O) of the healthcare provider organisation, contracted service provider (if applicable), HI Service operation (web service name), date time, HI Service message ID, batch ID (if applicable) version of the HI Service web service |
This test case is very similar to HI_010_008028 in UC.010 as it relates to retaining an audit trail of all healthcare identifiers associated with a record.
Test Case ID | HI_131_010041 |
---|---|
Test Objective | The software shall be capable of validating HPI-Is described in the HI Service system interface specification TECH.SIS.HI.15, 17, 31 or 33. |
How to evaluate | Perform an operation to validate the details of a Healthcare Provider using their HPI-I number in the HI Service |
This test case explains that CIS software shall be capable of providing a way to validate the HPI-I on the update screen as shown in the screenshot below. It is most likely that you would incorporate an HPI-I search every time the user’s details were changed and the user pressed a Save Changes or equivalent button. You may also want to have an additional Validate HPI-I button too.
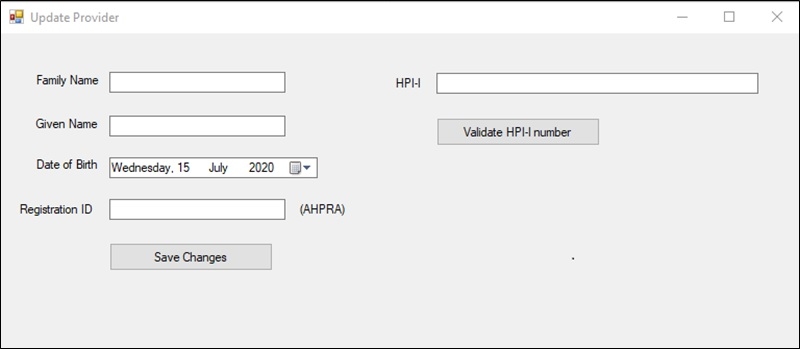
Test Case ID | HI_131_023502 |
---|---|
Test Objective | When the software attempts to validate a healthcare provider individual identifier (HPI-I) via the HI Service and the HI Service indicates the identifier is resolved or not active (e.g. retired, resolved, deactivated), the software shall perform the actions listed in the following table. Status of HPI-I Active: No action Retired: Warning Deactivated: Warning Resolved: Alert or warning (see below) When a healthcare provider individual identifier is validated and the HI Service returns a 'resolved’ status and a different healthcare provider individual identifier, the software shall not store that new healthcare provider identifier unless it can also be validated with the existing healthcare provider individual identifier demographic data in the local software. If the new healthcare provider individual identifier cannot be validated with the local healthcare provider individual identifier demographic data then an alert shall be raised so an operator can determine what action should be taken. The new healthcare provider individual identifier number and healthcare provider identifier status shall be stored in the healthcare provider record if the healthcare provider individual identifier can be validated using local healthcare provider demographic data. |
Important!
When the HPI-I status is “Resolved”, it isn’t returned as HPI-I Status, instead it is returned in serviceMessages with a code and reason
Code to below demonstrate the logic how to handle the resolved HPI-I
// Instantiate the client
var client = new ProviderSearchForProviderIndividualClient(
new Uri("https://www5.medicareaustralia.gov.au/cert/soap/services/"),
product,
user,
hpio,
signingCert,
tlsCert);
// Create the search request
var request = new searchForProviderIndividual()
{
familyName = txtFamilyName.Text,
hpiiNumber = HIQualifiers.HPIIQualifier + txtHPINumber.Text
};
try
{
// Invokes provider individual search.
var response = client.ProviderIndividualSearch(request);
var hpiSearchResult = response.searchForProviderIndividualResult;
if (hpiSearchResult.serviceMessages != null && hpiSearchResult.serviceMessages.serviceMessage[0].code == "WSE0134")
{
// Verify that the software revalidates the returned HPI-I with the local demographic data.
var returnedProvider = new Provider()
{
FamilyName = hpiSearchResult.familyName,
GiveName = hpiSearchResult.givenName,
DOB = hpiSearchResult.dateOfBirth,
// so on
}
// assume we have data in localProvider object.
if(verifDemographics(localProvider, returnedProvider))
{
// If the returned HPI-I can be validated successfully, ensure the returned HPI-I is stored in the provider record
}
else
{
// Verify that if the new HPI-I cannot be validated with the local provider demographic data then an alert shall be raised so an operator can determine what action should be taken.
DialogResult dialogResult = MessageBox.Show("The provider HPI-I cannot be validated with the name and registration information provided.", "HPI-I Warning", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
// Provide options to operator to determine what action should be taken.
}
}
switch(hpiSearchResult.status)
{
case "R":
MessageBox.Show("", "", MessageBoxButtons.OK, MessageBoxIcon.Warning);
break;
case "D":
MessageBox.Show("", "", MessageBoxButtons.OK, MessageBoxIcon.Warning);
break;
}
}
catch (FaultException fex)
{
string returnError = "";
MessageFault fault = fex.CreateMessageFault();
if (fault.HasDetail)
{
ServiceMessagesType error = fault.GetDetail<ServiceMessagesType>();
// Look at error details in here
if (error.serviceMessage.Length > 0)
returnError = error.serviceMessage[0].code + ": " + error.serviceMessage[0].reason;
}
string soapResponse = client.SoapMessages.SoapResponse;
}
Important!
The code provided above is for understating the test case logic, not to use as actual implementation in CIS software. Your software will require more advanced processing of error messages from the HI Service.
When local demographic data cannot be validated with returned HPI-I
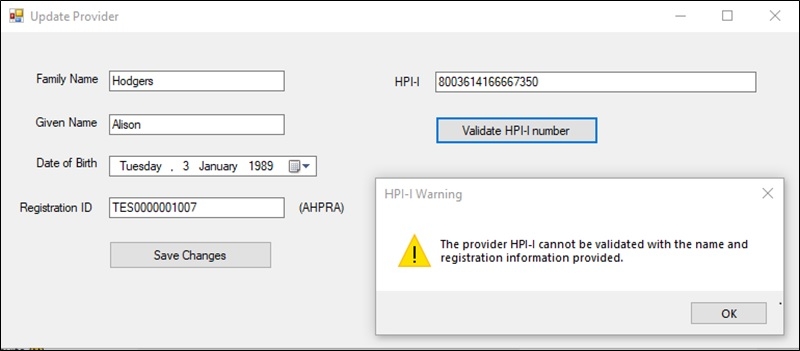
The following screenshot shows the SOAP Response when the HPI-I service message is showing an HPI-I which is resolved to a new HPI-I.
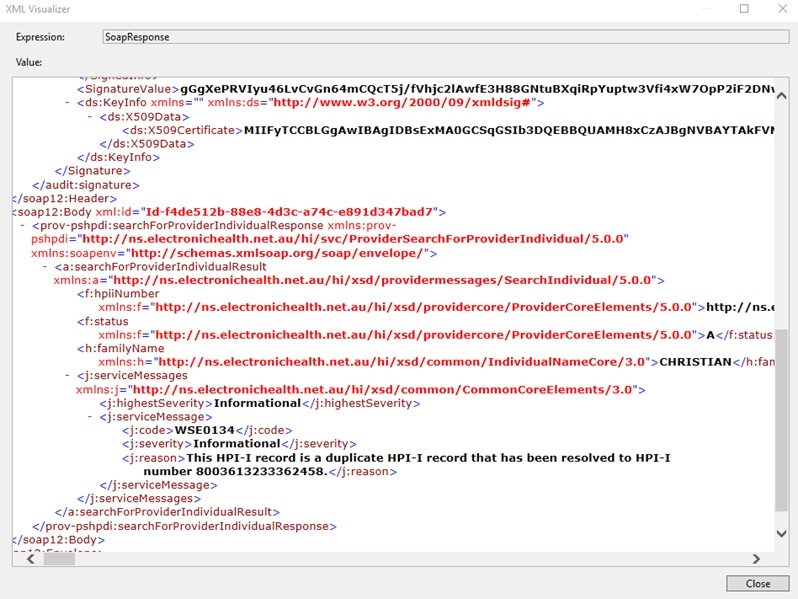
Test Case ID | HI_131_23503 |
---|---|
Test Objective | If the name associated with a HPI-I is changed then the software shall revalidate the HPI-I with the new demographic data. If the validation fails, then the software shall raise an alert against the HPI-I. |
How to evaluate | Update the family name that is associated with an active HPI-I. a. Verify that software allows the operator to revalidate the HPI-I to get the up-to-date HPI-I information. |
The following code demonstrates when the provider family name is changed (i.e. Marriage), it revalidates the HPI-I with the new demographics and raises an alert if the revalidation fails. Let’s assume we have method called validate HPI-I which makes a call to the HI Service. As mentioned above this could occur as part of the Save Changes (or similarly named) button.
private void UpdateProviderDetails(Provider provider)
{
// Before saving the provider details i.e. name, revalidate HPI-I
if(_hiService.ValidateHPI-I(provider)
{
// Save new information.
}
else
{
// Alert user
}
}
Conclusion
If you have followed the instructions clearly for the 2 HI Service Developer Guides, then you should now be ready to integrate this functionality more thoroughly into your product and undertake your HI Service Conformance Testing with the Digital Health Agency. Please contact [email protected] to book your testing. If you are connecting to the My Health Record system you are now also ready to progress to the first My Health Record Developer Guide: Find whether a My Health Record exists - My Health Record B2B Developer Guide 1.