Reading & Development: 2 hours
Quick Intro
When a patient has an appointment with a healthcare provider, and that healthcare provider is eligible for a Medicare rebate, a Medicare Benefits Schedule (MBS) clinical document is sent to Medicare. A similar situation occurs when a patient is dispensed a prescription which is listed on the Pharmaceutical Benefits Schedule (PBS). In this case a PBS clinical document is created. These 2 types of clinical documents flow from Medicare into a patient’s My Health Record unless they have modified their settings to turn this flow of data off.
An MBS document will not contain any clinically relevant information about the purpose of the visit, it’s use is primarily to identify the consultation type to allow a rebate payment to be made to the healthcare provider.
A PBS document does contain information about the medication being dispensed as this information is important for calculating rebates to the pharmacy.
It is useful to understand the content of these documents when implementing functionality to retrieve them.
In this guide, we will be accessing these 2 document types via the following 2 APIs;
1. PBS Items (GET)
2. MBS Items (GET)
Step 1: Get PBS Items (GET)
This API provides the ability to retrieve PBS details in the form of a bundle of ExplanationOfBenefit FHIR® resources from the My Health Record system. The API details are below.
Resource URI | [fqdn/fhir/v2.0.0]/ExplanationOfBenefit |
HTTP Method | GET (read) |
Request Headers | Authorization (OAuth Token), App-Id, App-Version, Platform-Version (optional) |
Request Parameters (searchParam) | patientreference - Logical identifier of the patient. (1..1)
Optional Request Parameters created – from date (yyyy-mm-dd) prefix with “ge” e.g., ge2016-05-20 ( 0..1) created - to date (yyyy-mm-dd) prefix with “le” e.g., le2016-05-20 (Cardinality: 0..1) _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir |
FHIR based resource reference |
View the API Specification Section 3.3 Medicare information for more specifics of the API use.
Step 2: PBS UI changes
1. Add new page called Medicare Information inside the project.
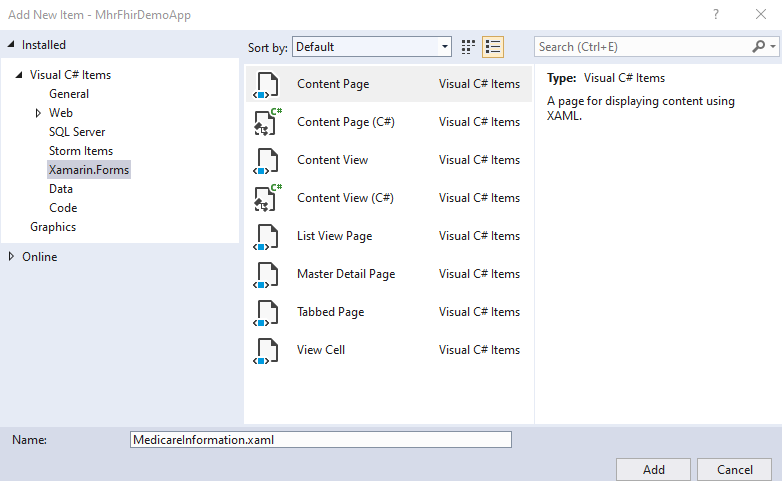
2. Add this new page into the Flyout Menu bar using the following code as highlighed.
<FlyoutItem FlyoutDisplayOptions="AsMultipleItems">
<ShellContent Route="LoginPage" ContentTemplate="{DataTemplate local:LoginPage}" />
<ShellContent Title="My Health Record" Icon="tab_about.png" Route="AboutPage" ContentTemplate="{DataTemplate local:AboutPage}" />
<ShellContent Title="Personal Health Summary" Route="HealthSummaryPage" ContentTemplate="{DataTemplate local:PersonalHealthSummaryPage}" />
<ShellContent Title="Prescription Dispense Allergy List" Route="PrescriptionDispensePage" ContentTemplate="{DataTemplate local:PrescriptionDispenseListPage}" />
<ShellContent Title="Generic Document Services List" Route="GenericDocumentServicesPage" ContentTemplate="{DataTemplate local:GenericDocumentServicesPage}" />
<ShellContent Title=”Medicare Information” Route=”MedicareInformationPage" ContentTemplate="{DataTemplate local:MedicareInformationPage}" />
</FlyoutItem>
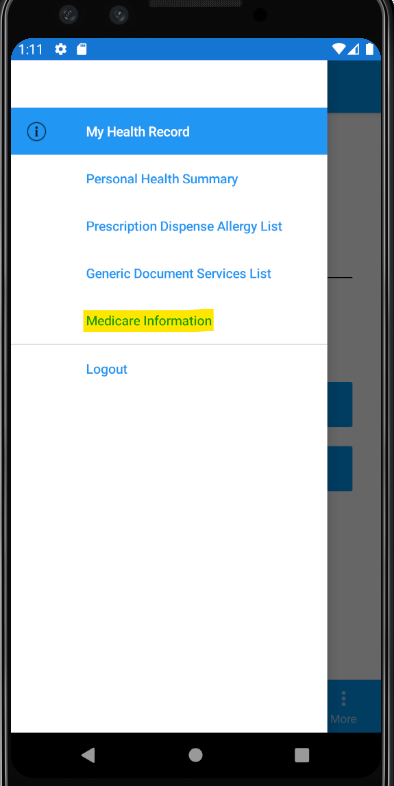
3. Add the UI Code (XAML) inside <ContentPage.Content> in the MedicareInformation.XAML file.
<StackLayout>
<Label Text="Medicare Information" Margin="0, 20, 0, 20" Style="{StaticResource PageHeader}"
VerticalOptions="Start"
HorizontalOptions="CenterAndExpand"
HorizontalTextAlignment="Center"/>
<BoxView HorizontalOptions="FillAndExpand" HeightRequest="1" Color="#222222" Margin="0, 0, 0, 20"/>
<Button Margin="0,10,0,0" Text="PBS Items" HorizontalOptions="Center"
x:Name="pbsItemsButton"
Clicked="pbsItemsButton_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
</StackLayout>
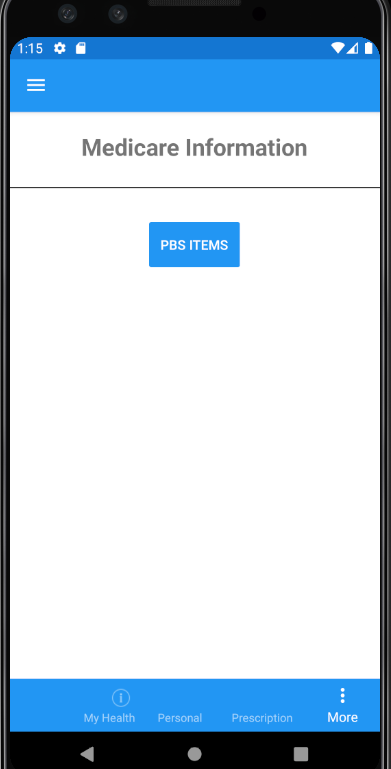
4. Add the methods inside the IMhrService interface and MhrService as show below.
a. IMhrService interface
Task<IRestResponse> GetPBSItemsList(string id, string fromDate = null, string toDate = null);
b. MhrService class
public async Task<IRestResponse> GetPBSItemsList(string id, string fromDate=null, string toDate=null)
{
RestRequest request = new RestRequest("ExplanationOfBenefit", Method.GET);
request.AddParameter("patientreference", id);
request.AddParameter("coverage.plan", "PBS");
// optional parameters
if (!string.IsNullOrEmpty(fromDate) && !string.IsNullOrEmpty(toDate))
{
request.AddParameter("created", "ge" + fromDate);
request.AddParameter("created", "le" + toDate);
}
// This method was already added in previous guide to add request headers such as authorization, app id, app version.
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
5. Add the following method on PBS Items button click event inside the MedicareInformationPage.xaml file.
private async void pbsItemsButton_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
string patientId = "1089887702";
string fromDate = "";
string toDate = "";
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetPBSItemsList(patientId, fromDate, toDate);
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
6. Run the application and test the PBS Items results. Some data from the bundle is provided below as an example of the response.
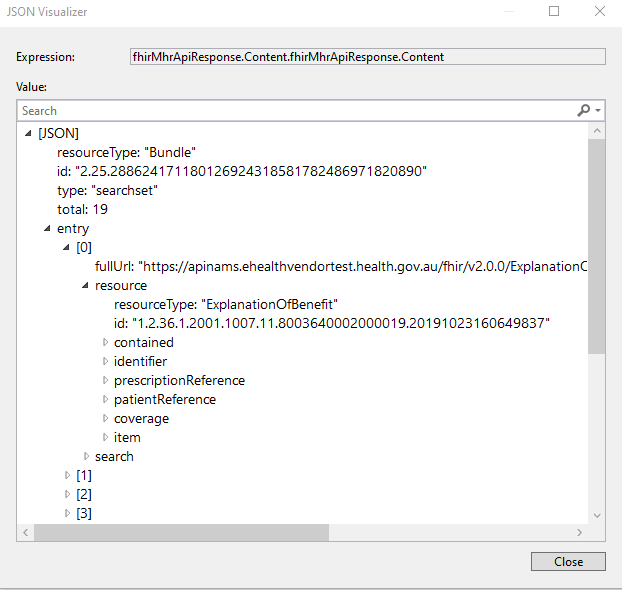
Step 3: Get MBS Items (GET)
This API provides the ability to retrieve MBS details for the individual and returns a bundle of ExplanationOfBenefit resources from the My Health Record system. You will note that it is the same as the PBS Items (GET) except for the coverage.plan has been changed to “MBS”. An example of this call has not been provided as it is self-explanatory and the previously created method could be modified to include an additional parameter allowing switch from PBS to MBS.
Resource URI | [fqdn/fhir/v2.0.0]/ExplanationOfBenefit |
HTTP Method | GET (read) |
Request Headers | Authorization (OAuth Token), App-Id, App-Version, Platform-Version (optional) |
Request Parameters (searchParam) | patientreference - Logical identifier of the patient. (Cardinality: 1..1)
Optional Request Parameters created – to date (yyyy-mm-dd) prefix with “ge” e.g., ge2016-05-20 (Cardinality: 0..1) created - to date (yyyy-mm-dd) prefix with “ge” e.g., ge2016-05-20 (Cardinality: 0..1) _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir |
FHIR based resource reference |
Conclusion
You should now have a working application which can access all of the main features of the My Health Record FHIR® gateway. We would love to hear feedback about your experience with these guides. Please send us an email on help@digitalhealth.gov.au.