Reading & Development: 2 hours
Quick Intro
In this guide, we will be accessing a patient’s medications and allergies contained in a Personal Health Summary. A Personal Health Summary is a specific type of clinical document which has been completed by the consumer, most often without the assistance of a healthcare professional. The complete specification for a Personal Health Summary can be found here if more information is needed.
Note
This is not the mechanism you would use to retrieve medications and allergies which have been added to the My Health Record by healthcare providers. This guide specifically relates to consumer-entered content of a Personal Health Summary.
Step 1: Medications (GET) API
This API provides the ability to retrieve consumer entered Medications from an individual’s Personal Health Summary document from the My Health Record system. The details of the API can be found in the table below.
View the API Specification Section 3.5.1 for more specifics of the API use.
Resource URI | [fqdn/fhir/v2.0.0]/MedicationStatement |
HTTP Method | GET (read) |
Request Headers | Authorization (OAuth Token ), App-Id, App-Version |
Request Parameters (searchParam) | Patient - Logical identifier of the patient. (1..1) _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir (to represent it as JSON). (0..1) |
FHIR Resource | http://hl7.org/fhir/2016May/medicationstatement.html |
We will now make some minor UI changes and then implement code to make the connection.
Step 2: UI changes
In the following steps we will add new UI page called Personal Health Summary and link to it in the Flyout menu bar.
1. Create a new content page called PersonalHealthSummaryPage.xaml.
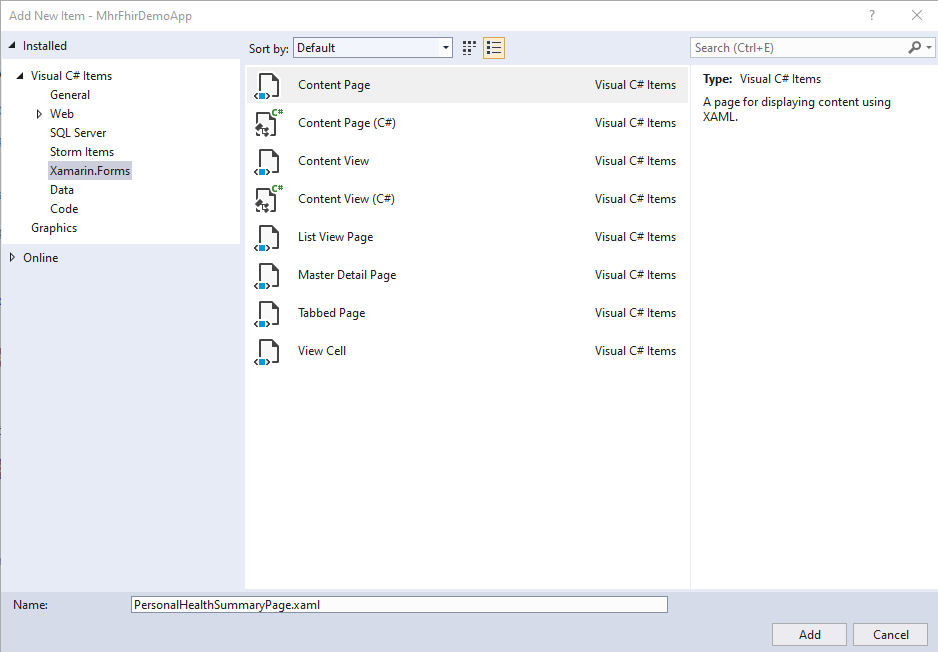
2. Add the following UI code between <ContentPage.Content> tags inside the PersonalHealthSummaryPage.xaml.
<StackLayout>
<Label Text="Personal Health Summary" Margin="0, 20, 0, 20" Style="{StaticResource PageHeader}"
VerticalOptions="Start"
HorizontalOptions="CenterAndExpand"
HorizontalTextAlignment="Center"/>
<BoxView HorizontalOptions="FillAndExpand" HeightRequest="1" Color="#222222" Margin="0, 0, 0, 20"/>
<Button Margin="0,10,0,0" Text="Medication List" HorizontalOptions="Center"
x:Name="medicationList"
Clicked="medicationList_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
</StackLayout>
3. Add the Personal Health Summary page link into the Flyout menu using the code below inside the AppShell.xaml file.
<ShellContent Title="Personal Health Summary" Route="HealthSummaryPage" ContentTemplate="{DataTemplate local:PersonalHealthSummaryPage}" />
The screenshots below demonstrate our UI changes.
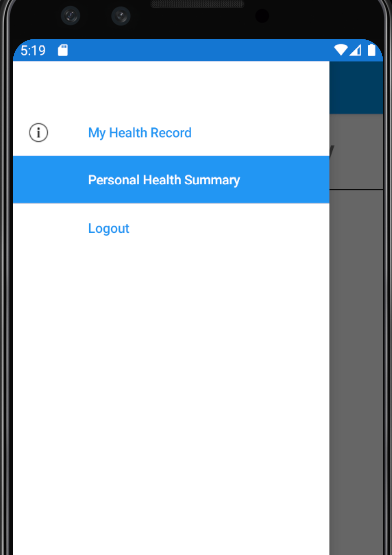
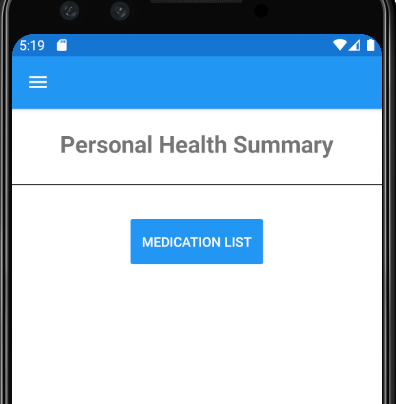
Step 3: Retrieving the medications
1. Add a new method inside the IMhrFhirService.
Task<IRestResponse> GetPHSMedicationList(string id);
2. Add an implementation method as per the code below inside the MhrFhirService class.
public async Task<IRestResponse> GetPHSMedicationList(string id)
{
RestRequest request = new RestRequest("MedicationStatement", Method.GET);
request.AddParameter("patient", id);
request.AddParameter("source._type", "Patient");
// This method was added in previous guide.
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
3. Add the following method on the Medication List button click event inside the partial class PersonalHealthSummaryPage.xaml.cs
private async void medicationList_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetPHSMedicationList("XXXXXXXXXX"); // Patient Id
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
Note
The Patient ID is different from the patient’s IHI and is retrieved via Patient Details (GET) and Record List (GET). You will remember us passing an empty string in the previous guide as the patient ID. To simplify the code example, we have hardcoded the Patient ID.
4. Run the application and test the Medication List. The response is provided below.
{
"resourceType":"Bundle",
"id":"1.2.36.1.2001.20191023164518248110001656455488275088606",
"type":"searchset",
"total":2,
"entry":[
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/MedicationStatement/1a34a699-9bb7-4ecf-8dd6-910832e0d079",
"resource":{
"resourceType":"MedicationStatement",
"id":"1a34a699-9bb7-4ecf-8dd6-910832e0d079",
"status":"active",
"medicationCodeableConcept":{
"text":"Novorapid Solostar"
},
"patient":{
"reference":"Patient/1089887702"
},
"informationSource":{
"reference":"Patient/1089887702"
},
"dateAsserted":"2019-10-23T16:45:00+11:00",
"reasonForUseCodeableConcept":{
"text":"Type 2 Diabetes Mellitus"
},
"note":[
{
"text":"insulin dependent"
}
],
"dosage":[
{
"text":"6 units, approx four times a day"
}
]
},
"search":{
"mode":"match"
}
},
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/MedicationStatement/5ee6070a-b5e6-4232-b62d-efed91e0dca1",
"resource":{
"resourceType":"MedicationStatement",
"id":"5ee6070a-b5e6-4232-b62d-efed91e0dca1",
"status":"active",
"medicationCodeableConcept":{
"text":"Glimepiride"
},
"patient":{
"reference":"Patient/1089887702"
},
"informationSource":{
"reference":"Patient/1089887702"
},
"dateAsserted":"2019-10-23T16:45:00+11:00",
"reasonForUseCodeableConcept":{
"text":"Type 2 Diabetes Mellitus"
},
"note":[
{
"text":"Taken before breakfast"
}
],
"dosage":[
{
"text":"1mg Tablet, once daily"
}
]
},
"search":{
"mode":"match"
}
}
]
}
Step 4: Allergies (GET) API
This API provides the ability to retrieve allergies and adverse reactions from the Personal Health Summary. See the table below for the API details.
Resource URI | [fqdn/fhir/v2.0.0]/AllergyIntolerance |
HTTP Method | GET (read) |
Request Headers | Authorization (Bearer OAuth Token), App-Id, App-Version |
Request Parameters (searchParam) | Patient - Logical identifier of the patient. (1..1) _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir (to represent it as JSON). |
FHIR Resource | http://hl7.org/fhir/2016May/allergyintolerance.html |
API Error Information | Use the Error Mapping guide supplied in the welcome pack folder. |
We will use the same UI page (Personal Health Summary) which was created in previous steps.
1. Add a new button called Allergies List under the Medication List button inside the PersonalHealthSummary.xaml file.
<Button Margin="0,10,0,0" Text="Allergies List" HorizontalOptions="Center"
x:Name="allergiesList"
Clicked="allergiesList_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
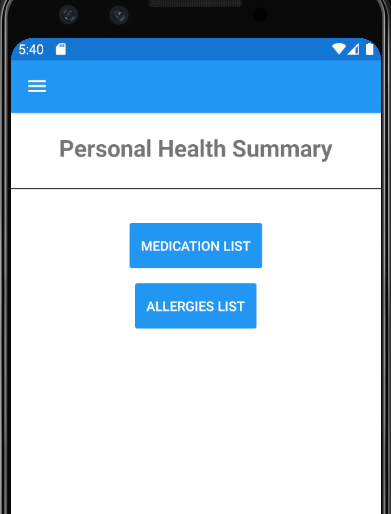
2. Add a new method inside the IMhrFhirService interface.
Task<IRestResponse> GetPHSAllergiesList(string id);
3 Add an implementation method inside the MhrFhirService class using the code below.
public async Task<IRestResponse> GetPHSAllergiesList(string id)
{
RestRequest request = new RestRequest("AllergyIntolerance", Method.GET);
request.AddParameter("patient", id);
request.AddParameter("reporter._type", "Patient");
// This method was already added in previous guide to add request headers such as authorization, //app id, app version.
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
4. Add the following method on the Allergies List button click event.
private async void allergiesList_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetPHSAllergiesList("XXXXXXXXXX"); // Patient Id
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
5. Before running the example code, supply the Patient ID into the GetPHSAllergiesList method.
6. Run the application and test the Allergies List button. A sample response is provided below.
{
"resourceType":"Bundle",
"id":"1.2.36.1.2001.20191023164518248110001656455488275088606",
"type":"searchset",
"total":2,
"entry":[
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/AllergyIntolerance/d2709202-365f-4387-b53c-fa0a73faba25",
"resource":{
"resourceType":"AllergyIntolerance",
"id":"d2709202-365f-4387-b53c-fa0a73faba25",
"substance":{
"text":"Amines"
},
"patient":{
"reference":"Patient/1089887702"
},
"recordedDate":"2019-10-23T16:45:00+11:00",
"recorder":{
"reference":"Patient/1089887702"
},
"reaction":[
{
"manifestation":[
{
"text":"Hives"
}
]
}
]
},
"search":{
"mode":"match"
}
},
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/AllergyIntolerance/f7cad730-5933-476b-9bd0-605f39f96055",
"resource":{
"resourceType":"AllergyIntolerance",
"id":"f7cad730-5933-476b-9bd0-605f39f96055",
"substance":{
"text":"Gluten"
},
"patient":{
"reference":"Patient/1089887702"
},
"recordedDate":"2019-10-23T16:45:00+11:00",
"recorder":{
"reference":"Patient/1089887702"
},
"reaction":[
{
"manifestation":[
{
"text":"Joint Pain"
},
{
"text":"Fatigue"
}
]
}
]
},
"search":{
"mode":"match"
}
}
]
}
Important
The Error Mapping document within the API specification package is an XLS which contains detailed information about error messages. Familiarise yourself with this file now.
Conclusion
You have now made your first calls to the My Health Record system and retrieved patient-entered information from the Personal Health Summary. In the next guides we will begin to retrieve information entered by healthcare providers.
View All | Back | Next: Clinical Document Services - My Health Record FHIR Gateway Developer Guide 5