Reading & Development: 2 hours
Overview
This guide will introduce accessing Prescription and Dispense List and Allergies List contained in a patient’s clinical document service. This differs from the previous guide where this information was accessed from the Personal Health Summary. As per FHIR® specifications you will notice that Prescriptions will be accessed using the MedicationOrder FHIR® resource, the Dispense Records via the MedicationDispense resource and allergies via AllergyIntolerance.
Step 1: Prescription List (GET)
This API provides the ability to retrieve prescription information for an individual from the My Health Record system and returns a bundle of MedicationOrder resources. A maximum of 99 resources are returned in the response.
Familiarise yourself with the API and parameters for the Prescription List below.
Resource URI | [fqdn/fhir/v2.0.0]/MedicationOrder |
---|---|
HTTP Method | GET (read) |
Request Headers | Authorization (OAuth Token), App-Id, App-Version |
Request Parameters (searchParam) | Patient - Logical identifier of the patient - 1..1 Datewritten - From Date (yyyy-mm-dd) – 0..1 Datewritten - To Date (yyyy-mm-dd) – 0..1 Add ‘ge’ or ‘le’ as prefix to indicate greater than or less than or equal to. _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir |
FHIR based resource reference |
View the API Specification Section 3.6 for a more in depth understanding.
In the next few steps, we will modify our UI and add the code-behind logic to retrieve the Prescription and Dispense List returned as JSON.
Step 1.1. Add a new UI page
1. Create a new content page called PrescriptionDispenseListPage.xaml.
2. Add the following UI code between <ContentPage.Content> tags inside the PrescriptionDispenseListPage.xaml file.
<StackLayout>
<Label Text="Clinical Document Services" Margin="0, 20, 0, 20" Style="{StaticResource PageHeader}"
VerticalOptions="Start"
HorizontalOptions="CenterAndExpand"
HorizontalTextAlignment="Center"/>
<BoxView HorizontalOptions="FillAndExpand" HeightRequest="1" Color="#222222" Margin="0, 0, 0, 20"/>
<Button Margin="0,10,0,0" Text="Prescription List" HorizontalOptions="Center"
x:Name="prescriptionList"
Clicked="prescriptionList_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
</StackLayout>
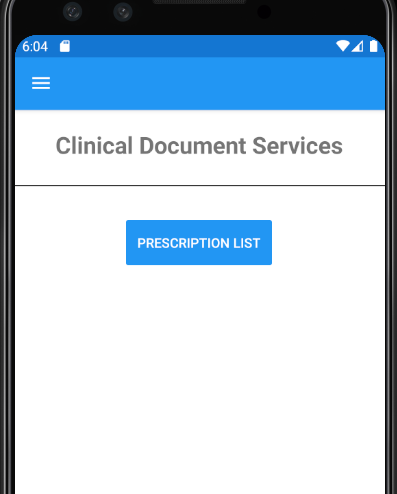
3. Add this new page into the Flyout Menu bar using the following code inside the AppShell.xaml file.
<ShellContent Title="Prescription Dispense Allergy List" Route="PrescriptionDispensePage" ContentTemplate="{DataTemplate local:PrescriptionDispenseListPage}" />
Step 1.2. Add code to retrieve Prescriptions
1. Add a new method inside the IMhrFhirService
Task<IRestResponse> GetPrescriptionList(string id, string fromDate, string toDate);
2. Add an implementation method as per the code below inside the MhrFhirService class
public async Task<IRestResponse> GetPrescriptionList(string id, string fromDate, string toDate)
{
RestRequest request = new RestRequest("MedicationOrder", Method.GET);
request.AddParameter("patient", id);
request.AddParameter("datewritten", "ge"+fromDate);
request.AddParameter("datewritten", "le"+toDate);
// This method was already added in the previous guide to add request headers such as authorisation, app id, app version.
await AddStandardHeaders(request); // Added in previous guide.
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
3. Add the following method on Prescription List button click event inside the partial class PrescriptionDispenseListPage.xaml.cs. Hard-coded date values have been used for this example.
private async void prescriptionList_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
string fromDate = "2010-01-01";
string toDate = "2020-12-31";
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetPrescriptionList("1089887702", fromDate, toDate); // Patient Id
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
4. Run the application and test the Prescriptions List.
By using the Visual Studio debugger, JSON data can be seen in the JSON visualizer as illustrated below.
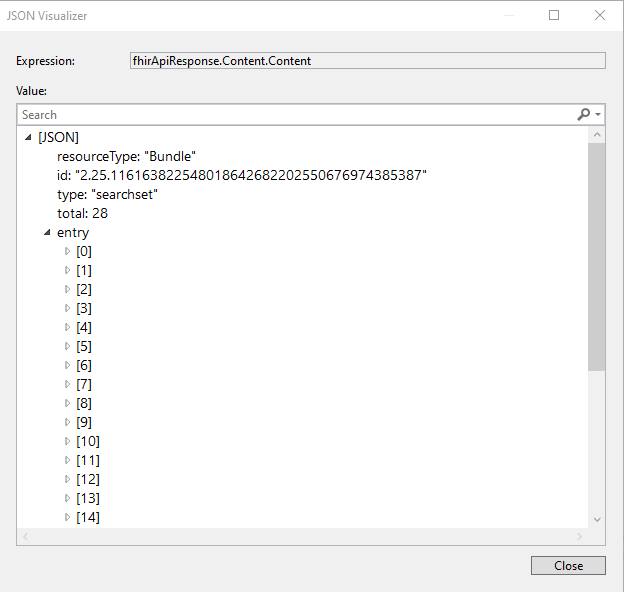
The complete JSON object has not been expanded to ensure readability of this guide.
Step 2: Dispense List (GET)
The following code for the Dispense List issimilar. As per the API specifics below a bundle of MedicationDispense resources will be received.
Resource URI | [fqdn/fhir/v2.0.0]/MedicationDispense |
---|---|
HTTP Method
| GET (read) |
Request Headers | Authorization (OAuth Token ), App-Id, App-Version |
Request Parameters (searchParam) | Patient - Logical identifier of the patient. (1..1) whenhandedover - From Date is a search criteria to select the documents whose start date is after the specific period. The date format must be prefixed with the value of ‘ge’ to indicate the created date must be greater-than or equal to the provided date. Example: ge2016-05-20 (1..1) whenhandedover - To Date is a search criteria to select the documents whose start date is after the specific period. (1..1). The date format must be prefixed with the value of ‘ge’ to indicate the created date must be less-than or equal to the provided date. Example: le2018-07-14 _include - Include the prescription reference in the response. Allowed Parameter Value: MedicationDispense:authorizingPrescription (0..1) _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir (0..1) |
FHIR based resource reference | http://hl7.org/fhir/2016May/medicationdispense.html |
Step 2.1. Add a button called Dispense List underneath the Prescription List button inside the PrescriptionDispenseListPage.XAML file.
<Button Margin="0,10,0,0" Text="Dispense List" HorizontalOptions="Center"
x:Name="dispenseList"
Clicked="dispenseList_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
Step 2.2. Add a new method inside the IMhrFhirService interface.
Task<IRestResponse> GetDispenseList(string id, string fromDate, string toDate);
Step 2.3. Add an implementation method as per the code below inside the MhrFhirService class created in previous guides.
public async Task<IRestResponse> GetDispenseList(string id, string fromDate, string toDate)
{
RestRequest request = new RestRequest("MedicationDispense", Method.GET);
request.AddParameter("patient", id);
request.AddParameter("whenhandedover", "ge" + fromDate);
request.AddParameter("whenhandedover", "le" + toDate);
// This method was already added in previous guide to add request headers such as authorization, app id, app version.
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
Step 2.4. Add the following method on the Dispense List button click event added in previous step inside the partial class PrescriptionDispenseListPage.xaml.cs
private async void dispenseList_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
string fromDate = "2010-01-01";
string toDate = "2020-12-31";
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetDispenseList("1089887702", fromDate, toDate); // Patient Id
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
Step 2.5. Run the application and test the Dispense List.
By using the Visual Studio debugger, the JSON Visualizer can display JSON as illustrated below. The MedicationDispense entry has been partially expanded.
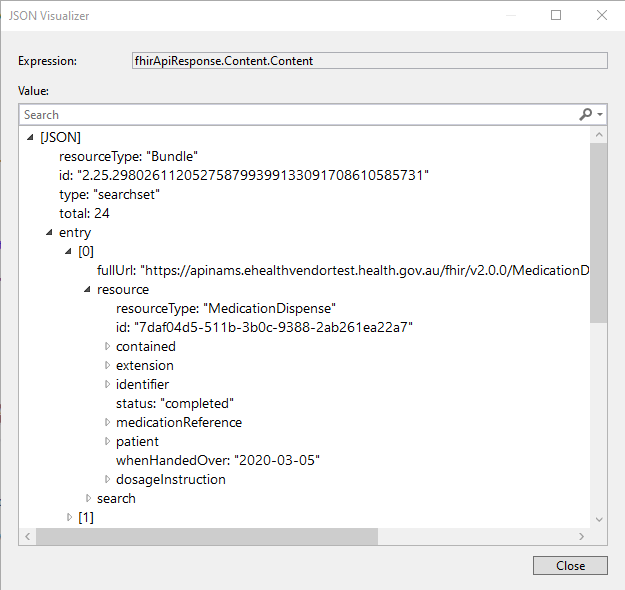
public async Task<IRestResponse> GetAllergyIntoleranceList(string id)
{
RestRequest request = new RestRequest("AllergyIntolerance", Method.GET);
request.AddParameter("patient", id);
request.AddParameter("reporter._type", "Practitioner");
// This method was already added in previous guide to add request headers such as authorization, app id, app version.
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
Step 3: Get Allergies List (SHS) (GET)
This API provides the ability to retrieve an individual’s allergies and adverse reactions information and returns a bundle of AllergyIntolerance resources from the most recent Shared Health Summary (SHS) document stored in the consumer’s My Health Record. If you are unfamiliar with the concept of SHS, please take a moment to read about its purpose here.
The same AllergyIntolerance API in the previous guide will be accessed here, however in this instance, reporter._type “Practitioner” will be used instead of “Patient”.
Resource URI | [fqdn/fhir/v2.0.0]/AllergyIntolerance |
---|---|
HTTP Method | GET (read) |
Request Headers | Authorization (Bearer OAuth Token ), App-Id, App-Version, Platform-Version (optional) |
Request Parameters (searchParam) | Patient - Logical identifier of the patient. (Cardinality: 1..1) _format - The suggested values are application/xml+fhir (to represent it as XML) OR application/json+fhir (to represent it as JSON). (Cardinality: 0..1) |
FHIR based resource reference |
The following code is to retrieve the patient’s AllergyIntolerance information from the My Health Record System. There are many similarities to the previous code and you could leverage the method already created for the Personal Health Summary AllergyIntolerance request by simply adding a parameter to parse for reporter._type.
The example below creates a new method.
public async Task<IRestResponse> GetAllergyIntoleranceList(string id)
{
RestRequest request = new RestRequest("AllergyIntolerance", Method.GET);
request.AddParameter("patient", id);
request.AddParameter("reporter._type", "Practitioner");
// This method was already added in previous guide to add request headers such as authorization, app id, app version.
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
Conclusion
The sample application can now access medications and allergies information added to the My Health Record by healthcare providers. This builds on previously developed functionality to retrieved Patient Health Summary information. The next guide will provide details on accessing clinical documents directly by using the Generic Document Service.
View All | Back | Next: Generic Document Services - My Health Record FHIR® Gateway Developer Guide 6