Introduction
This guide will introduce the steps to retrieve:
- Patient Details
- A list of associated persons (i.e. dependants)
The UI will be modified by adding 2 buttons to access this data as per the screenshot below.
Important
You will note that the My Health Record logo has been used. When using the My Health Record logo you should be sure to conform to all branding guidelines. You can access logo and branding resources here.
Pre-requisites
To begin this guide you should have completed the previous two guides:
- My Health Record FHIR® Mobile Gateway - Registration and Overview
- My Health Record FHIR® Mobile Gateway - Environment Setup and Authentication
The token received from completing MyGov authentication in the previous guide will be used to access the patient’s My Health Record.
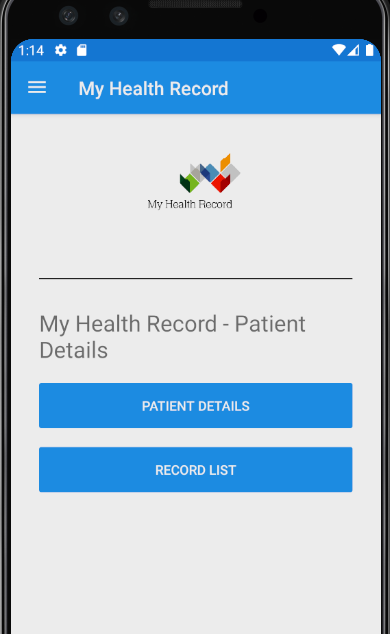
Step 1: Get Patient Details
Now that you have the authentication token securely saved, you can make subsequent HTTP calls.
We will now make an HTTP Get request to retrieve an individual’s demographic details from the My Health Record system. The table below provides you with the details of the GET request along with the applicable FHIR® Resource information on the HL7 website.
Resource URI | [fqdn/fhir/v2.0.0]/Patient/[id] |
HTTP Method | GET (read) |
Request Headers | Authorization (OAuth Token ), App-Id, App-Version |
FHIR Resource | http://hl7.org/fhir/2016May/patient.html |
More Information
You can view the specifications for Get Patient Details from the API Specification. As at v2.0.0 Get Patient Details is described in section 3.2.2. Familiarise yourself with this section of the document now (7 pages).
We will now use the existing About page to display the 2 buttons as per the screenshot above.
Open the About.xaml file and replace UI code with the following code inside the <ContentPage> tag.
<ContentPage.Resources>
<ResourceDictionary>
<Color x:Key="Accent">#ffffff</Color>
</ResourceDictionary>
</ContentPage.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<StackLayout BackgroundColor="{StaticResource Accent}" VerticalOptions="FillAndExpand" HorizontalOptions="Fill">
<StackLayout Orientation="Horizontal" HorizontalOptions="Center" VerticalOptions="Center">
<ContentView Padding="0,40,0,40" VerticalOptions="FillAndExpand">
<Image Source="MyHealthRecord_logo.png" VerticalOptions="Center" HeightRequest="64" />
</ContentView>
</StackLayout>
</StackLayout>
<ScrollView Grid.Row="1">
<StackLayout Orientation="Vertical" Padding="30,24,30,24" Spacing="10">
<BoxView HorizontalOptions="FillAndExpand" HeightRequest="1" Color="#222222" Margin="0, 0, 0, 20"/>
<Label Text="My Health Record - Patient Details" FontSize="Title"/>
<Button Margin="0,10,0,0" Text="Patient Details"
x:Name="patientDetails"
Clicked="PatientDetails_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
</StackLayout>
</ScrollView>
</Grid>
Note
The My Health Record logo has been included in the Resources/Drawable folder of the Android Project.
This application uses RestSharp library to make HTTP calls. The following steps will update your code to make the Get Patient Details call. Some of the code added here will be common and used for several sections of these guides.
Step 1.1. Install RestSharp using the following command in the Package Manager Console
Install-Package RestSharp
Step 1.2. Create an interface with the name IMhrFhirService and add the following code.
using RestSharp;
public interface IMhrFhirService
{
Task<IRestResponse> GetPatientDetails(string id);
Step 1.3. Create a new class called MhrFhirService and add the following code.
using RestSharp;
using System.Threading.Tasks;
using Xamarin.Essentials;
public class MhrFhirService : IMhrFhirService
{
private readonly RestClient _httpClient = null;
public MhrFhirService()
{
_httpClient = new RestClient($"{App.FhirApiEndPoint}/{App.FhirApiVersion}");
}
public async Task<IRestResponse> GetPatientDetails(string id)
{
RestRequest request;
if (string.IsNullOrEmpty(id))
{
request = new RestRequest("Patient", Method.GET);
}
else
{
request = new RestRequest("Patient/{id}", Method.GET);
request.AddUrlSegment("id", id);
}
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = await _httpClient.ExecuteAsync(request);
return fhirApiResponse;
}
public async Task AddStandardHeaders(RestRequest request)
{
//Optional
request.AddHeader("Content-Type", "application/json+fhir");
request.AddHeader("Accept", "application/json+fhir");
//Mandatory
string token = await SecureStorage.GetAsync(App.AuthTokenKey);
request.AddHeader("Authorization", "Bearer " + token);
request.AddHeader("App-Id", App.ClientId);
request.AddHeader("App-Version", App.AppVersion);
}
}
Step 1.4. Add the MHRFHIRService class into DependencyService inside the App.xaml.cs file as highlighted in the code below.
public App()
{
InitializeComponent();
DependencyService.Register<MockDataStore>();
DependencyService.Register<MhrFhirService>();
MainPage = new AppShell();
}
Step 1.5. Add the following code to the Get Patient button click event inside the About.xaml.cs file.
private async void PatientDetails_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
// If id is not passed, it returns logged-in patient details.
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetPatientDetails("");
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
In the GetPatientDetails method, we are passing an empty string which will use the logged-in patient details by default. In the next section, we will check the GetRecordList HTTP call which retrieves the patient and related personal details with the patients’ ids.
Note
Patient Id and IHI are two different identifiers.
Step 1.6. Run the application, login again and test the Patient Details Page About.xaml.
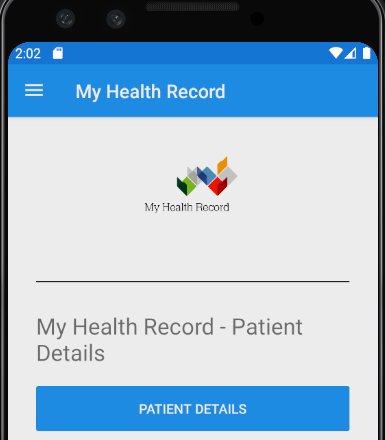
If the HTTP response status is OK, it will return the patient details JSON as per HL7 FHIR® specifications. The blocks below contain the JSON response, familiarise yourself with this structure.
string json = fhirMhrApiResponse.Content;
{
"resourceType":"Bundle",
"id":"43deeaa1-f2d2-418c-b563-ac225ecf0293",
"type":"searchset",
"total":4,
"entry":[
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/Patient/1089887702",
"resource":{
"resourceType":"Patient",
"id":"1089887702",
"extension":[
{
"url":"http://hl7.org.au/fhir/StructureDefinition/indigenous-status",
"valueCoding":{
"system":"http://meteor.aihw.gov.au/content/index.phtml/itemId/602543#Codes",
"code":"9",
"display":"Not stated/inadequately described"
}
}
],
"identifier":[
{
"type":{
"coding":[
{
"system":"http://hl7.org/fhir/v2/0203",
"code":"NI",
"display":"National unique individual identifier"
}
],
"text":"IHI"
},
"system":"http://ns.electronichealth.net.au/id/hi/ihi/1.0",
"value":"8003608666888243"
}
],
"active":true,
"name":[
{
"family":[
"MOSES"
],
"given":[
"SHAWN"
]
}
],
"gender":"female",
"birthDate":"1979-08-13"
},
"search":{
"mode":"match"
}
},
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/Patient/1089887727",
"resource":{
"resourceType":"Patient",
"id":"1089887727",
"extension":[
{
"url":"http://hl7.org.au/fhir/StructureDefinition/indigenous-status",
"valueCoding":{
"system":"http://meteor.aihw.gov.au/content/index.phtml/itemId/602543#Codes",
"code":"4",
"display":"Neither Aboriginal nor Torres Strait Islander origin"
}
}
],
"identifier":[
{
"type":{
"coding":[
{
"system":"http://hl7.org/fhir/v2/0203",
"code":"NI",
"display":"National unique individual identifier"
}
],
"text":"IHI"
},
"system":"http://ns.electronichealth.net.au/id/hi/ihi/1.0",
"value":"8003608666888250"
}
],
"active":true,
"name":[
{
"family":[
"MOSES"
],
"given":[
"MARCELLA"
]
}
],
"gender":"female",
"birthDate":"2010-12-19"
},
"search":{
"mode":"match"
}
},
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/Patient/1089887729",
"resource":{
"resourceType":"Patient",
"id":"1089887729",
"extension":[
{
"url":"http://hl7.org.au/fhir/StructureDefinition/indigenous-status",
"valueCoding":{
"system":"http://meteor.aihw.gov.au/content/index.phtml/itemId/602543#Codes",
"code":"9",
"display":"Not stated/inadequately described"
}
}
],
"identifier":[
{
"type":{
"coding":[
{
"system":"http://hl7.org/fhir/v2/0203",
"code":"NI",
"display":"National unique individual identifier"
}
],
"text":"IHI"
},
"system":"http://ns.electronichealth.net.au/id/hi/ihi/1.0",
"value":"8003608333562551"
}
],
"active":true,
"name":[
{
"family":[
"CRIPPS"
],
"given":[
"ERNIE"
]
}
],
"gender":"male",
"birthDate":"1977-02-28"
},
"search":{
"mode":"match"
}
},
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/Patient/1089887728",
"resource":{
"resourceType":"Patient",
"id":"1089887728",
"extension":[
{
"url":"http://hl7.org.au/fhir/StructureDefinition/indigenous-status",
"valueCoding":{
"system":"http://meteor.aihw.gov.au/content/index.phtml/itemId/602543#Codes",
"code":"9",
"display":"Not stated/inadequately described"
}
}
],
"identifier":[
{
"type":{
"coding":[
{
"system":"http://hl7.org/fhir/v2/0203",
"code":"NI",
"display":"National unique individual identifier"
}
],
"text":"IHI"
},
"system":"http://ns.electronichealth.net.au/id/hi/ihi/1.0",
"value":"8003608166895276"
}
],
"active":true,
"name":[
{
"family":[
"LOCKYER"
],
"given":[
"PERRY"
]
}
],
"gender":"male",
"birthDate":"1963-11-26"
},
"search":{
"mode":"match"
}
}
]
}
Important
Please take a moment to note the location of the Patient id in the JSON above. The id for the first patient returned is “1089887702”. Yours will be different as you will be using different test data.
Step 1.7. To parse the JSON content and serialize it into an object, we have a library available from the HL7 FHIR® resources. You may wish to use the following example to parse the JSON content into the FHIR® POCO classes.
a. Install the following library using Package Manager Console.
Install-Package Hl7.Fhir.STU3
b. Modify the following method as per the code below to parse the JSON content (returned as a string).
private async void PatientDetails_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetPatientDetails("");
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
FhirJsonParser parser = new FhirJsonParser();
var patientBundle = (Bundle) parser.Parse(json);
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
Step 2: Get Record List (GET)
The Get Record List API provides the ability to retrieve the list of records the individual is permitted to access and returns a bundle containing the RelatedPerson resource for each accessible record. These persons might be children or maybe a person under the care of the user.
More Information
More information about authorised representatives can be found here. Familiarise yourself with this content now.
You can view the specifications for Get Record List from the API Specification. As at v2.0.0 Get Record List is described in section 3.2.1. Familiarise yourself with this section of the document now (3 pages).
The request details are outlined in the table below along with the HL7 FHIR® Resource link.
Resource URI HTTP Method | [fqdn/fhir/v2.0.0]/RelatedPerson GET (search) |
Request Headers | Authorization, App-Id, App-Version, Platform-Version |
Request Parameters (searchParam) | _format |
FHIR Based resource | http://hl7.org/fhir/2016May/relatedperson.html |
In the following steps, you will add the code to make an HTTP call to Get Record List.
Step 2.1. Add a new button called Record List inside the About.xaml file below the Patient Details button as shown in the code and the screenshot provided earlier.
<Button Margin="0,10,0,0" Text="Record List"
x:Name="btnRecordList"
Clicked="btnRecordList_Clicked"
BackgroundColor="{StaticResource Primary}"
TextColor="White" />
Step 2.2. Add the interface method inside the IMhrFhirService created in the previous steps.
Task<IRestResponse> GetRecordList();
Step 2.3. Add the service method inside the MhrFhirService class to make the HTTP call for Get Record List using the code below.
public async Task<IRestResponse> GetRecordList()
{
var request = new RestRequest("RelatedPerson", Method.GET);
await AddStandardHeaders(request);
IRestResponse fhirApiResponse = _httpClient.Execute(request);
return fhirApiResponse;
}
Step 2.4. Add the following code to the Record List button click event inside the About.xaml.cs file.
private async void btnRecordList_Clicked(object sender, EventArgs e)
{
IMhrFhirService mhrFhirService = DependencyService.Get<MhrFhirService>();
IRestResponse fhirMhrApiResponse = await mhrFhirService.GetRecordList();
if (fhirMhrApiResponse.StatusCode == HttpStatusCode.OK)
{
string json = fhirMhrApiResponse.Content;
}
else
{
string errorDesc = fhirMhrApiResponse.StatusDescription;
}
}
Step 2.5. Run the application and test the Get Record List functionality.
The screenshot below shows the JSON content using the Visual Studio Text Visualizer in Debug Mode.
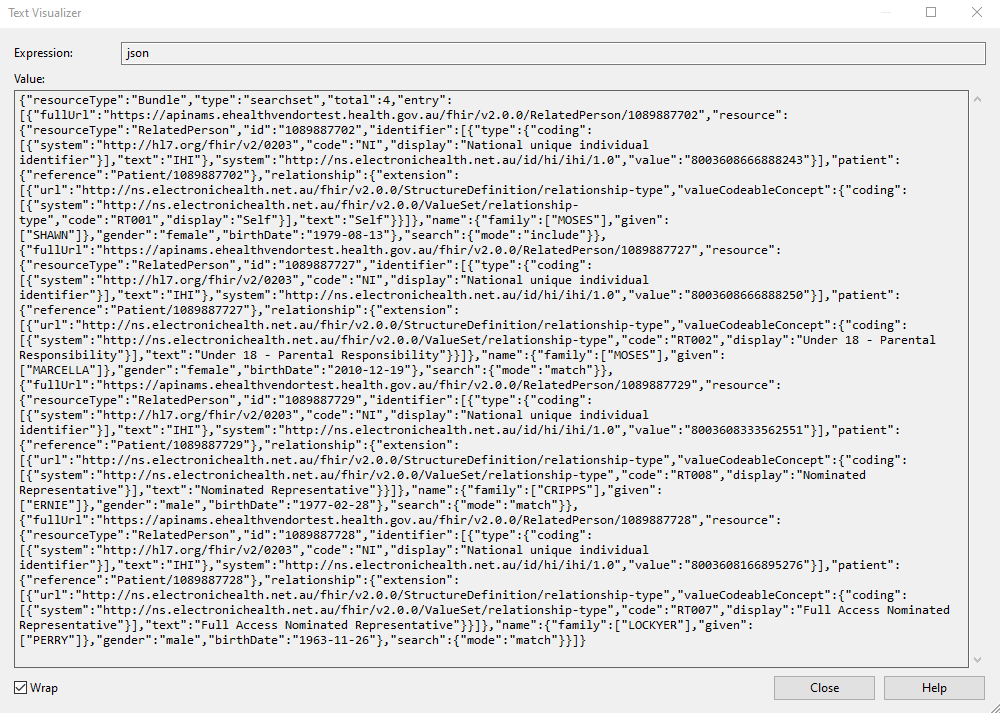
Familiarise yourself with the response.
Note
To present the JSON content neatly below and remove redundancies we have reduced the contents to two records of Related Person.
{
"resourceType":"Bundle",
"type":"searchset",
"total":4,
"entry":[
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/RelatedPerson/1089887702",
"resource":{
"resourceType":"RelatedPerson",
"id":"1089887702",
"identifier":[
{
"type":{
"coding":[
{
"system":"http://hl7.org/fhir/v2/0203",
"code":"NI",
"display":"National unique individual identifier"
}
],
"text":"IHI"
},
"system":"http://ns.electronichealth.net.au/id/hi/ihi/1.0",
"value":"8003608666888243"
}
],
"patient":{
"reference":"Patient/1089887702"
},
"relationship":{
"extension":[
{
"url":"http://ns.electronichealth.net.au/fhir/v2.0.0/StructureDefinition/relationship-type",
"valueCodeableConcept":{
"coding":[
{
"system":"http://ns.electronichealth.net.au/fhir/v2.0.0/ValueSet/relationship-type",
"code":"RT001",
"display":"Self"
}
],
"text":"Self"
}
}
]
},
"name":{
"family":[
"MOSES"
],
"given":[
"SHAWN"
]
},
"gender":"female",
"birthDate":"1979-08-13"
},
"search":{
"mode":"include"
}
},
{
"fullUrl":"https://apinams.svt.gw.myhealthrecord.gov.au/fhir/v2.0.0/RelatedPerson/1089887727",
"resource":{
"resourceType":"RelatedPerson",
"id":"1089887727",
"identifier":[
{
"type":{
"coding":[
{
"system":"http://hl7.org/fhir/v2/0203",
"code":"NI",
"display":"National unique individual identifier"
}
],
"text":"IHI"
},
"system":"http://ns.electronichealth.net.au/id/hi/ihi/1.0",
"value":"8003608666888250"
}
],
"patient":{
"reference":"Patient/1089887727"
},
"relationship":{
"extension":[
{
"url":"http://ns.electronichealth.net.au/fhir/v2.0.0/StructureDefinition/relationship-type",
"valueCodeableConcept":{
"coding":[
{
"system":"http://ns.electronichealth.net.au/fhir/v2.0.0/ValueSet/relationship-type",
"code":"RT002",
"display":"Under 18 - Parental Responsibility"
}
],
"text":"Under 18 - Parental Responsibility"
}
}
]
},
"name":{
"family":[
"MOSES"
],
"given":[
"MARCELLA"
]
},
"gender":"female",
"birthDate":"2010-12-19"
},
"search":{
"mode":"match"
}
},
]
}
Conclusion
This developer guide introduced My Health Record FHIR® APIs Get Patient Details and Record List (Related Person) of a healthcare recipient using the same demo mobile app created in the previous guide. In the following guides we will begin to retrieve clinical information.
View All | Back | Next: Personal Health Summary - My Health Record FHIR Gateway Developer Guide 4